User Search v2
Auth0 allows you, as an administrator, to search for users using Lucene Query Syntax.
This document provides sample queries and demonstrates how you can search for users. We also suggest that you refer to Query Syntax for more examples of query string syntax.
Search for users using the Management API
You can also search for users using the Management API. The easiest way to do this is by making use of the API Explorer. This technique is discussed briefly below, but please note that the Auth0 Management API is a REST API, so you can make API calls using anything that can make HTTP requests, or by using one of the Auth0 SDKs.
In order to make requests to the Management API, you will need a token. Please refer to Access Tokens for the Management API for more information.
Search using the API Explorer
To search users using the Management API Explorer, go to the Users section and then select List or search users. Scroll down to the q
parameter. You can use any query string which uses the query syntax in this field.
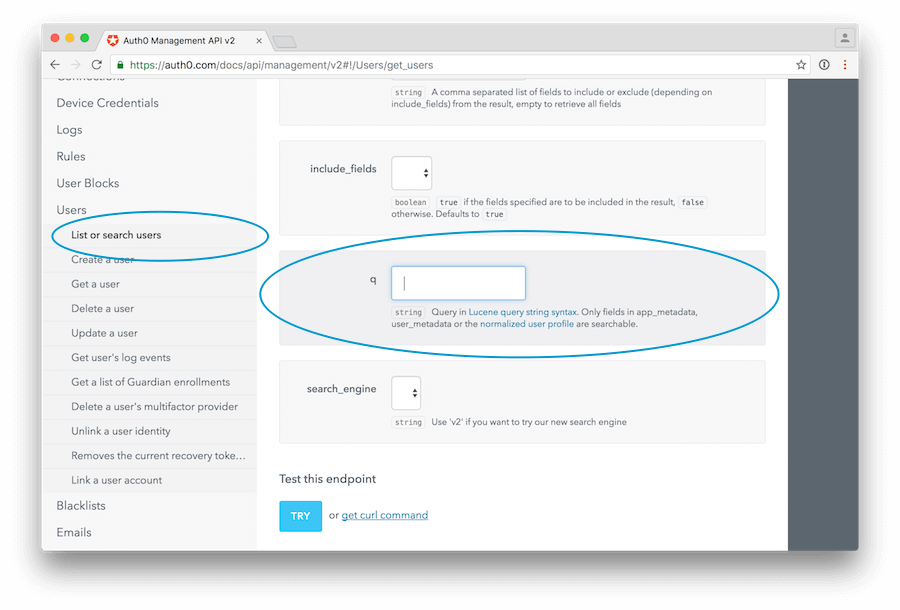
Sorting search results
To sort the list of users returned from the Management API, you can make use of the sort
parameter. Use the format field:order
for the value of the sort
field, where field
is the name of the field you want to sort by, and order
can be 1
for ascending and -1
for descending. For example, to sort users in ascending order by the created_at
field you can pass the value of created_at:1
for the sort
parameter. Sorting by app_metadata
or user_metadata
is not supported.
For more information on the sort
and other parameters, please refer to the Management API Explorer documentation.
Exact matching and tokenization
Because of the manner in which ElasticSearch handles tokenization on +
and -
, unexpected results can occur when searching by some fields. For example, when searching for a user whose name
is jane
(name:"jane"
), the results will be both for jane
and jane-doe
, because both of these contain the exact search term that you used. The difference may not affect some searches, but it will affect others, and provide unanticipated results.
You can solve this problem either by using structured JSON in your metadata, or by using the raw subfield.
Using the raw subfield
If you wish to avoid the potential pitfalls of analyzed data and search for an exact match to your term - an exact string comparison - then for some fields you can use the raw
subfield, which will be not_analyzed
.
So, in the example name.raw:"jane"
, the user data for jane
would match, but jane-doe
would not.
The fields that support raw
subfield queries are:
identities.connection
identities.provider
identities.user_id
email
phone_number
family_name
given_name
username
name
nickname
Example queries
Below are some example queries to illustrate the kinds of queries that are possible using the Management API V2.
Use Case | Query |
---|---|
Search for all users whose name contains "john" | name:"john" |
Search all users whose name is exactly "john" | name.raw:"john" |
Search for all user names starting with "john" | name:john* |
Search for user names that start with "john" and end with "smith" | name:john*smith |
Search for all users whose email is exactly "john@contoso.com" | email.raw:"john@contoso.com" |
Search for all users whose email is exactly "john@contoso.com" or "mary@contoso.com" using OR |
email.raw:("john@contoso.com" OR "mary@contoso.com") |
Search for users without verified email | email_verified:false OR NOT _exists_:email_verified |
Search for users who have the user_metadata field named name with the value of "John Doe" |
user_metadata.name:"John Doe" |
Search for users from a specific connection or provider | identities.provider:"google-oauth2" |
Search for all users that have never logged in | (NOT _exists_:logins_count OR logins_count:0) |
Search for all users who logged in before 2015 | last_login:[* TO 2014-12-31] |
Fuzziness: Search for terms that are similar to, but not exactly like, jhn |
name:jhn~ |
All users with more than 100 logins | logins_count:>100 |
Logins count >= 100 and <= 200 | logins_count:[100 TO 200] |
Logins count >= 100 | logins_count:[100 TO *] |
Logins count > 100 and < 200 | logins_count:{100 TO 200} |
Example request
Below is an example request for searching all users whose email is exactly "john@contoso.com".
curl --request GET \
--url 'https://{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2' \
--header 'authorization: Bearer ACCESS_TOKEN'
Was this helpful?
var client = new RestClient("https://{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2");
var request = new RestRequest(Method.GET);
request.AddHeader("authorization", "Bearer ACCESS_TOKEN");
IRestResponse response = client.Execute(request);
Was this helpful?
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2"
req, _ := http.NewRequest("GET", url, nil)
req.Header.Add("authorization", "Bearer ACCESS_TOKEN")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
Was this helpful?
HttpResponse<String> response = Unirest.get("https://{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2")
.header("authorization", "Bearer ACCESS_TOKEN")
.asString();
Was this helpful?
var axios = require("axios").default;
var options = {
method: 'GET',
url: 'https://{yourDomain}/api/v2/users',
params: {q: 'email.raw:"john@contoso.com"', search_engine: 'v2'},
headers: {authorization: 'Bearer ACCESS_TOKEN'}
};
axios.request(options).then(function (response) {
console.log(response.data);
}).catch(function (error) {
console.error(error);
});
Was this helpful?
#import <Foundation/Foundation.h>
NSDictionary *headers = @{ @"authorization": @"Bearer ACCESS_TOKEN" };
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"GET"];
[request setAllHTTPHeaderFields:headers];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"%@", httpResponse);
}
}];
[dataTask resume];
Was this helpful?
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => [
"authorization: Bearer ACCESS_TOKEN"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Was this helpful?
import http.client
conn = http.client.HTTPSConnection("")
headers = { 'authorization': "Bearer ACCESS_TOKEN" }
conn.request("GET", "/{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2", headers=headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Was this helpful?
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
request["authorization"] = 'Bearer ACCESS_TOKEN'
response = http.request(request)
puts response.read_body
Was this helpful?
import Foundation
let headers = ["authorization": "Bearer ACCESS_TOKEN"]
let request = NSMutableURLRequest(url: NSURL(string: "https://{yourDomain}/api/v2/users?q=email.raw%3A%22john%40contoso.com%22&search_engine=v2")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "GET"
request.allHTTPHeaderFields = headers
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if (error != nil) {
print(error)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()
Was this helpful?