The Auth0 team released a new major version of the Auth0 NextJS SDK which incorporates much of the received feedback from our v1, including support for NextJS middleware, changes in the API, first-class testing support, optimizations on the Front End package, and lots more.
This article will cover some of the most significant changes, but for a complete set, plus indications on how to migrate your current project to the new SDK, I recommend following the official migration guide.
NextJS Middleware Support
The introduction of NextJS middleware in v12 was a big step for the framework that allows you to run code before a request is completed.
This support has been highly requested by the community, and the benefits can be summarized in two aspects: simplification and performance improvement.
It's a lot easier to add authentication code
Prior to middleware support, each page that required authentication would need to add a snippet of code to tell NextJS only to allow access to authenticated users.
In v2, all that spread-out code can be rewritten at a single location by using middleware like the following:
// middleware.js import { withMiddlewareAuthRequired } from '@auth0/nextjs-auth0/middleware'; export default withMiddlewareAuthRequired(); export const config = { matcher: '/user/:path*', }
With that simple configuration, NextJS will run the middleware
withMiddlewareAuthRequired
when the URL matches the given matcher
expression. The matcher expressions are a feature of NextJS, they are very customizable, and you can read more information about them on NextJS's middleware docs.Performance
Since middleware in NodeJS runs on the NextJS Edge Runtime, validating user sessions at that level gives an immediate performance boost by simply reducing the latency for users. It is no longer required for the request to be sent to the origin server and back if the user is not authenticated.
In the image below, you can see how the NextJS Middleware Architecture plays a role in performance by being closer to the user's location.
A Delightful New Declarative Routing API
From v1, the SDK has been deeply customizable, but users often have to write full request handlers to access some of the lower-level features of the SDK. With the new declarative API for creating request handlers, this can be done simply and beautifully through the API.
Take a look at the following snippets of code.
Using SDK v1
export default handleAuth({ signup: async function signup(req, res) { try { await handleLogin(req, res, { authorizationParams: { screen_hint: 'signup' } }); } catch (error) { res.status(error.status || 400).end(error.message); } }, invite: async function invite(req, res) { try { await handleLogin(req, res, { authorizationParams: { invitation: req.query.invitation } }); } catch (error) { res.status(error.status || 400).end(error.message); } }, 'login-with-google': async function loginWithGoogle(req, res) { try { await handleLogin(req, res, { authorizationParams: { connection: 'google' } }); } catch (error) { res.status(error.status || 400).end(error.message); } }, 'refresh-profile': async function refreshProfile(req, res) { try { await handleProfile(req, res, { refetch: true }); } catch (error) { res.status(error.status || 400).end(error.message); } }, }
Using SDK v2
export default handleAuth({ signup: loginHandler({ authorizationParams: { login_hint: 'signup' } }), invite: loginHandler({ authorizationParams: (req) => { invitation: req.query.invitation } }), 'login-with-google': loginHandler({ authorizationParams: { connection: 'google' } }), 'refresh-profile': profileHandler({ refetch: true }), }
Isn't that much nicer?
First Class Testing Support
Testing routes that require login has always been a complex problem for developers. The new SDK release includes built-in support for seeding the session cookie on your tests to make testing authenticated pages a breeze.
Instead of having to manage secrets in tests, visit third-party websites or mock complex internals of authentication libraries, users can use the new testing functionality built right into the SDK.
Consider the following code:
import { generateSessionCookie } from '@auth0/nextjs-auth0/testing'; test('should visit profile page', async () => { const cookie = await generateSessionCookie(session, config); const res = visit('/profile', { headers: { cookies: `appSession=${cookie}` } }); expect(res.status).toBe(200); })
The code above uses Auth0 NextJS SDK to generate a session cookie which is later sent as part of the
visit
request.If the session is not provided, the request would respond with a
403 Forbidden
as the URL is protected against unauthenticated requests.And LOTS more…
But that is not all. The new SDK offers you:
- New session lifecycle and
method.updateUser
- New package export profile to support React Server Components.
- Improved API Docs.
- Improved Error Handling.
- Lighter Front-End binary.
- Support for custom Logout Options.
- Easier options for managing cookie size.
Summary
The Auth0 NextJS SDK v2 introduced some pretty neat changes that will ultimately provide a better developer and end-user experience.
For those projects migrating from the SDK v1, I recommend reading the official migration guide.
Thanks for reading!
About the author
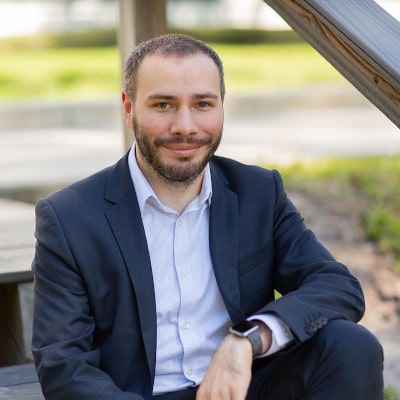
Juan Cruz Martinez
Staff Developer Advocate