We’re excited to announce the released version 2.16.0 to our Auth0 React Native SDK. Major features we delivered in this release include:
- Expo support
- Hooks API
- Secure Credentials Manager
Support for Expo
Expo is a popular framework that adds a suite of features to React Native apps. We have added support for this tooling to make it much easier to work with our SDK in conjunction with Expo, as it provides a number of benefits when used to build React Native apps.
We added this support in response to growing customer demand. We received various feedback across our channels that indicated to us that this would be a valuable addition to our SDK. In an excellent demonstration of the power of open-source software, our implementation actually started life as a pull request from the community, which was able to review, tidy up, and merge into our codebase for the benefit of others.
Given Expo’s growing popularity among the React Native community, it was clear this was an opportunity to provide first-class support in the SDK. To that end, we also now provide an Expo-specific quickstart in addition to our standard React Native quickstart so that developers can get up and running even quicker.
New React Hooks API
This release adds support for using the SDK using React Hooks. This was primarily driven by our own desire to modernize the SDK and provide an API that felt more natural to modern React developers. React Hooks have been in React Native 0.59 (released around March 2019). Our hooks wrap our pre-existing WebAuth classes, so the developer can choose to use Hooks or to continue using the provided classes should they wish.
In addition, we updated our React Native quickstart to use the new Hooks API by default.
How to use the new Hooks API
Previously, the developer could instantiate a class and use the properties and methods provided to implement authentication into their apps.
import Auth0 from 'react-native-auth0'; import jwt_decode from 'jwt-decode'; const auth0 = new Auth0({ domain: 'YOUR_AUTH0_DOMAIN', clientId: 'YOUR_AUTH0_CLIENT_ID', }); const App = () => { let [user, setUser] = useState(null); const onLogin = () => { auth0.webAuth .authorize({ scope: 'openid profile email' }) .then(credentials => { const payload = jwt_decode(credentials.idToken); setUser(payload) }) .catch(error => console.log(error)); }; return ( <View> {!user && <Button onPress={onLogin} title="Log in" />} {user && <Text>Logged in as {user.name}</Text>} </View > ); } export default App;
Now, the developer can use Hooks to implement login, providing an idiomatic way to implement reactive code in a React Native app using function components:
// App.js import {Auth0Provider} from 'react-native-auth0'; const App = () => { return ( <Auth0Provider domain="YOUR_AUTH0_DOMAIN" clientId="YOUR_AUTH0_CLIENT_ID"> {/* YOUR APP */} </Auth0Provider> ); };
// Component.js const Component = () => { const {authorize, user} = useAuth0(); const login = async () => { await authorize(); }; return ( <View> {!user && <Button onPress={login} title="Log in" />} {user && <Text>Logged in as {user.name}</Text>} </View> ); };
Secure Credentials Manager
Up until this release, the developer was in charge of storing and managing the tokens that were returned to the app from Auth0 without any assumptions from the SDK as to how and where tokens were stored. Now, we provide a built-in secure credentials manager that automatically stores these tokens.
This was another feature that was driven by community asks but one that makes a lot of sense if we’re interested in making things as frictionless for the developer as possible. We had a number of requests across our channels for this, and we’re pleased to be able to provide it as a first-class addition to the SDK
This mechanism uses the thoroughly tested credentials manager instances provided by Auth0.Swift and Auth0.Android.
Automatically refreshes the access token when it expires.
Automatically stores and clears tokens when using the Hooks API.
Support for platform-specific Biometric Authentication
Summary
The Auth0 React Native SDK v2.16.0 improves both developer and the user experience. On the one hand, it provides flexibility for developers to be up and running in less time. On the other hand, it provides a more secure credentials manager that can automatically manage tokens.
For further reading: Introducing the Auth0 Flutter SDK
About the author
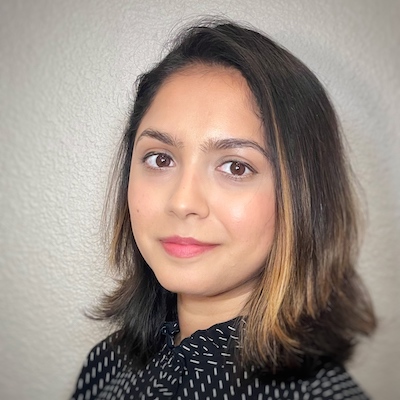
Ruchita Shah
Senior Product Marketing Manager, Customer Identity