Authenticate with Private Key JWT
Before you start
You need to create a new application in Auth0 Dashboard or convert an existing application before you continue. To learn more, read Configure Private Key JWT Authentication.
You need to complete two steps when authenticating with private_key_jwt:
Build the client assertion. This assertion is a JWT signed by the private key when you generated the key pair. To learn how to generate a key pair, read Configure Private Key JWT Authentication.
Use the assertion to authenticate against Auth0.
Build the assertion
You can use one of Auth0’s SDKs to build an assertion automatically for you. If you don’t use our SDKs, you will need to construct the assertion yourself.
The assertion is a JSON Web Token (JWT) that should contain the following properties and claims:
Header
alg
: The algorithm used to sign the assertion. The algorithm must match the algorithm specified when you created your application credential.kid
: (optional) The Auth0 generatedkid
of the credential. Thekid
is created when you created the credential.
Payload
iss
: Your application's Client ID. You can find this value in your application settings under Auth0 Dashboard > Applications > Applications and select the Settings tab.sub
: Your application's Client ID. You also can find this value in your application settings. You can find this value in your application settings under Auth0 Dashboard > Applications > Applications and select the Settings tab.aud
: The URL of the Auth0 tenant or custom domain that receives the assertion. For example:https://{yourTenant}.auth0.com/
. Include the trailing slash.iat
(optional) andexp
: Issued At and Expiration claims set to the correct timestamps. The client assertion is a one-time use token, and we recommend the shortest possible expiry time. Auth0 supports a maximum of 5 minutes for the lifetime of a token.jti
: A unique claim ID created by the client. We recommend using the Universally Unique Identifier (UUID) format.
The token must then be signed with the private key you generated when you created or configured your application for Private Key JWT Authentication. To learn how, review the JSON Web Token specification.
We recommend you construct the token using standard tooling or third party libraries that support this functionality out of the box, rather than implementing from scratch yourself. To learn more about supporting libraries, read the listing at JWT.io.
Example
In the example below, the Node.js script uses a jose package to generate the assertion:
const { SignJWT } = require('jose')
const crypto = require("crypto");
const uuid = require("uuid");
async function main() {
const privateKeyPEM = crypto.createPrivateKey(/**
Read the content of your private key here. We recommend to store your private key
in a secure infrastructure.
*/);
const jwt = await new SignJWT({})
.setProtectedHeader({
alg: 'RS256', // or RS384 or PS256
kid: '(OPTIONAL) KID_GENERATED_BY_AUTH0'
})
.setIssuedAt()
.setIssuer('CLIENT_ID')
.setSubject('CLIENT_ID')
.setAudience('https://YOUR_TENANT.auth0.com/') // or your CUSTOM_DOMAIN
.setExpirationTime('1m')
.setJti(uuid.v4())
.sign(privateKeyPEM);
console.log(jwt)
}
main();
Was this helpful?
Example client assertion signed with a private key:
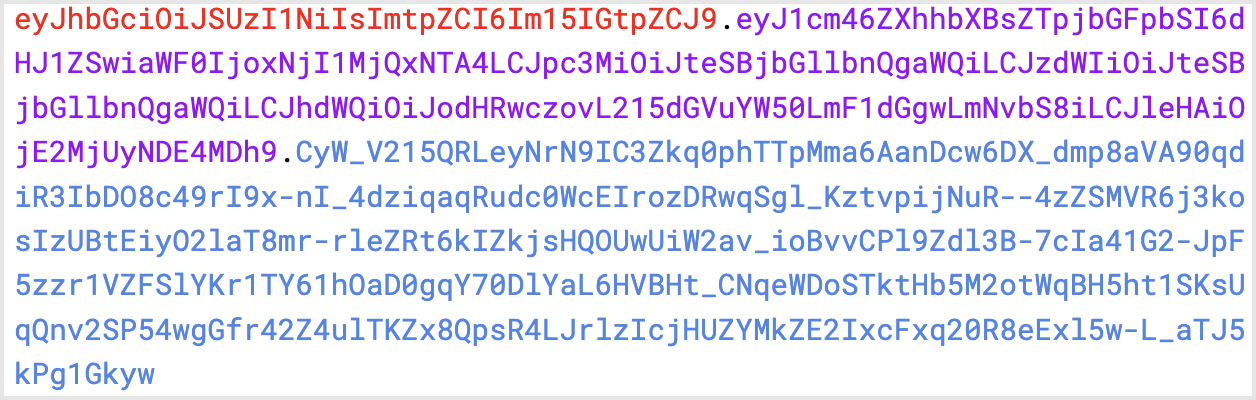
Corresponds to:
{
"alg": "RS256",
"kid": "my kid"
}
{
"iat": 1626684584,
"iss": "my client id",
"sub": "my client id",
"aud": "https://mytenant.auth0.com/",
"exp": 1626684644,
"jti": "e4dc8ed1-b108-4901-8bbc-c07a791817e7"
}
Was this helpful?
After you generate the JWT with the required information, you are ready to authenticate your application against Auth0.
Exchange assertion for access tokens
To exchange the JWT assertion for an access token, call the Authentication API token endpoint with the following parameters:
$client_assertion
: JWT assertion$resource_server_identifier
: resource server identifier. To learn more, read Register APIs.
curl --location --request POST 'https://$tenant/oauth/token' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'grant_type=client_credentials' \
--data-urlencode 'client_assertion_type=urn:ietf:params:oauth:client-assertion-type:jwt-bearer' \
--data-urlencode 'client_assertion=$client_assertion' \
--data-urlencode 'audience=$resource_server_idenifier'
Was this helpful?
Supported endpoints
In addition to the https://$tenant/oauth/token endpoint, the following Auth0 Authentication API endpoints support private_key_jwt
authentication for configured applications:
Assertion limits
The maximum length of the JWT assertion is 2048 bytes.
Claims within the assertion have the following limits:
iss
: 64 characterssub
: 64 charactersjti
: 64 charactersalg
: 16 characters