Auth0.swift
Overview
Swift SDK for iOS, macOS, tvOS, and watchOS that enables you to seamlessly integrate Auth0 into your apps. Add login and logout, store credentials securely, and access user information.
See the GitHub repository
Migrating from v1? Check the Migration Guide
Documentation
Quickstart: Shows how to integrate Auth0.swift into an iOS / macOS app from scratch.
Sample App: A complete, running iOS / macOS app you can try.
Examples: Explains how to use most features.
API Documentation: Documentation auto-generated from the code comments that explains all the available features.
FAQ: Answers some common questions about Auth0.swift.
Auth0 Documentation: Explore our docs site and learn more about Auth0.
Getting started
Requirements
iOS 13.0+ / macOS 11.0+ / tvOS 13.0+ / watchOS 7.0+
Xcode 14.x
Swift 5.7+
Installation
Swift Package Manager
Open the following menu item in Xcode:
File > Add Packages...
In the Search or Enter Package URL search box enter this URL:
https://github.com/auth0/Auth0.swift
Was this helpful?
Then, select the dependency rule and press Add Package.
Cocoapods
Add the following line to your Podfile
:
pod 'Auth0', '~> 2.0'
Was this helpful?
Then, run pod install
.
Carthage
Add the following line to your Cartfile
:
github "auth0/Auth0.swift" ~> 2.0
Was this helpful?
Then, run carthage bootstrap --use-xcframeworks
.
Configure the SDK
Go to the Auth0 Dashboard and create a new Native application.
Auth0.swift needs the Client ID and Domain of the Auth0 application to communicate with Auth0. You can find these details in the Settings page of your Auth0 application. If you are using a custom domain, use the value of your custom domain instead of the value from the Settings page.
Configure Client ID and Domain with a plist
Create a plist
file named Auth0.plist
in your app bundle with the following content:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>ClientId</key>
<string>{yourAuth0ClientId}</string>
<key>Domain</key>
<string>{yourAuth0Domain}</string>
</dict>
</plist>
Was this helpful?
Configure Client ID and Domain programmatically
For Web Auth
Auth0
.webAuth(clientId: "{yourAuth0ClientID}", domain: "{yourAuth0Domain}")
// ...
Was this helpful?
For the Authentication API client
Auth0
.authentication(clientId: "{yourAuth0ClientID}", domain: "{yourAuth0Domain}")
// ...
Was this helpful?
For the Management API client (Users)
Auth0
.users(token: credentials.accessToken, domain: "{yourAuth0Domain}")
// ...
Was this helpful?
Configure Web Auth (iOS / macOS)
Configure callback URL and logout URL
The callback URL and logout URL are the URLs that Auth0 invokes to redirect back to your application. Auth0 invokes the callback URL after authenticating the user, and the logout URL after removing the session cookie.
Since the callback URL and logout URL can be manipulated, you will need to add your URLs to the Allowed Callback URLs and Allowed Logout URLs fields in the Settings page of your Auth0 application. This will enable Auth0 to recognize these URLs as valid. If the callback URL and logout URL are not set, users will be unable to log in and out of the application and will get an error.
Go to the Settings page of your Auth0 application and add the corresponding URL to Allowed Callback URLs and Allowed Logout URLs, according to the platform of your application. If you are using a custom domain, replace {yourAuth0Domain}
with the value of your custom domain instead of the value from the Settings page.
iOS
{yourBundleIdentifier}://{yourAuth0Domain}/ios/{yourBundleIdentifier}/callback
Was this helpful?
macOS
{yourBundleIdentifier}://{yourAuth0Domain}/macos/{yourBundleIdentifier}/callback
Was this helpful?
For example, if your iOS bundle identifier was com.example.MyApp
and your Auth0 Domain was example.us.auth0.com
, then this value would be:
com.example.MyApp://example.us.auth0.com/ios/com.example.MyApp/callback
Was this helpful?
Configure custom URL scheme
In Xcode, go to the Info tab of your app target settings. In the URL Types section, click the + button to add a new entry. There, enter auth0
into the Identifier field and $(PRODUCT_BUNDLE_IDENTIFIER)
into the URL Schemes field.
This registers your bundle identifier as a custom URL scheme, so the callback URL and logout URL can reach your app.
Web Auth login (iOS / macOS)
Import the Auth0
module in the file where you want to present the login page.
import Auth0
Was this helpful?
Then, present the Universal Login page in the action of your Login button.
Auth0
.webAuth()
.start { result in
switch result {
case .success(let credentials):
print("Obtained credentials: \(credentials)")
case .failure(let error):
print("Failed with: \(error)")
}
}
Was this helpful?
Using async/await
do {
let credentials = try await Auth0.webAuth().start()
print("Obtained credentials: \(credentials)")
} catch {
print("Failed with: \(error)")
}
Was this helpful?
Using Combine
Auth0
.webAuth()
.start()
.sink(receiveCompletion: { completion in
if case .failure(let error) = completion {
print("Failed with: \(error)")
}
}, receiveValue: { credentials in
print("Obtained credentials: \(credentials)")
})
.store(in: &cancellables)
Was this helpful?
Web Auth logout (iOS / macOS)
Logging the user out involves clearing the Universal Login session cookie and then deleting the user's credentials from your application.
Call the clearSession()
method in the action of your Logout button. Once the session cookie has been cleared, delete the user's credentials.
Auth0
.webAuth()
.clearSession { result in
switch result {
case .success:
print("Session cookie cleared")
// Delete credentials
case .failure(let error):
print("Failed with: \(error)")
}
}
Was this helpful?
Using async/await
do {
try await Auth0.webAuth().clearSession()
print("Session cookie cleared")
// Delete credentials
} catch {
print("Failed with: \(error)")
}
Was this helpful?
Using Combine
Auth0
.webAuth()
.clearSession()
.sink(receiveCompletion: { completion in
switch completion {
case .finished:
print("Session cookie cleared")
// Delete credentials
case .failure(let error):
print("Failed with: \(error)")
}
}, receiveValue: {})
.store(in: &cancellables)
Was this helpful?
SSO alert box (iOS / macOS)
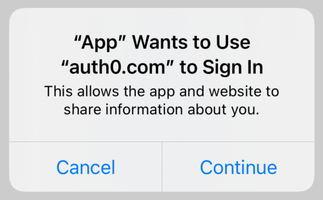
Check the FAQ for more information about the alert box that pops up by default when using Web Auth.
Next steps
Learn about most features in Examples:
Store credentials: Store the user's credentials securely in the Keychain.
Check for stored credentials: Check if the user is already logged in when your app starts up.
Retrieve stored credentials: Get the user's credentials from the Keychain, automatically renewing them if they have expired.
Clear stored credentials: Delete the user's credentials to complete the logout process.
Retrieve user information: Get the latest user information from the
/userinfo
endpoint.