iOS / macOS
This guide demonstrates how to integrate Auth0 with any new or existing iOS / macOS app using the Auth0.swift SDK. We recommend that you log in to follow this quickstart with examples configured for your account.
I want to integrate with my app
15 minutesI want to explore a sample app
2 minutesGet a sample configured with your account settings or check it out on Github.
Configure Auth0
You will need a Native Auth0 application. If you don’t have a Native Auth0 application already, create one before continuing. Avoid using other application types, as they have different configurations and may cause errors.
Configure the callback and logout URLs
The callback and logout URLs are the URLs that Auth0 invokes to redirect back to your app. Auth0 invokes the callback URL after authenticating the user, and the logout URL after removing the session cookie.
If the callback and logout URLs are not set, users will be unable to log in and out of the app and will get an error.
Go to the settings page of your Auth0 application and add the following URLs to Allowed Callback URLs and Allowed Logout URLs, depending on the platform of your app. If you have a custom domain, use this instead of the value from the settings page.
iOS
https://{yourDomain}/ios/YOUR_BUNDLE_IDENTIFIER/callback,
YOUR_BUNDLE_IDENTIFIER://{yourDomain}/ios/YOUR_BUNDLE_IDENTIFIER/callback
Was this helpful?
macOS
https://{yourDomain}/macos/YOUR_BUNDLE_IDENTIFIER/callback,
YOUR_BUNDLE_IDENTIFIER://{yourDomain}/macos/YOUR_BUNDLE_IDENTIFIER/callback
Was this helpful?
For example, if your iOS bundle identifier were com.example.MyApp
and your Auth0 domain were example.us.auth0.com
, then this value would be:
https://example.us.auth0.com/ios/com.example.MyApp/callback,
com.example.MyApp://example.us.auth0.com/ios/com.example.MyApp/callback
Was this helpful?
Configure the associated domain
Configure the Team ID and bundle identifier
Scroll to the end of the settings page of your Auth0 application and open Advanced Settings > Device Settings. In the iOS section, set Team ID to your Apple Team ID, and App ID to your app's bundle identifier.
This will add your app to your Auth0 tenant's apple-app-site-association
file.
Add the associated domain capability
In Xcode, go to the Signing and Capabilities tab of your app's target settings, and press the + Capability button. Then select Associated Domains.
Next, add the following entry under Associated Domains:
webcredentials:{yourDomain}
Was this helpful?
If you have a custom domain, use this instead of the Auth0 domain from the settings page.
Install the SDK
Add the Auth0.swift SDK to your project. The library will make requests to the Auth0 Authentication and Management APIs.
Using the Swift Package Manager
Open the following menu item in Xcode:
File > Add Package Dependencies...
In the Search or Enter Package URL search box enter this URL:
https://github.com/auth0/Auth0.swift
Was this helpful?
Then, select the dependency rule and press Add Package..
Using Cocoapods
Add the following line to your Podfile
:
pod 'Auth0', '~> 2.0'
Was this helpful?
Then, run pod install
.
Using Carthage
Add the following line to your Cartfile
:
github "auth0/Auth0.swift" ~> 2.0
Was this helpful?
Then, run carthage bootstrap --use-xcframeworks
.
Configure the SDK
The Auth0.swift SDK needs the Client ID and domain of the Auth0 application to communicate with Auth0. You can find these details in the settings page of your Auth0 application. If you are using a custom domain, use the value of your custom domain instead of the value from the settings page.
Create a plist
file named Auth0.plist
in your app bundle with the following content:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>ClientId</key>
<string>{yourClientId}</string>
<key>Domain</key>
<string>{yourDomain}</string>
</dict>
</plist>
Was this helpful?
Checkpoint
Now that you have configured Auth0.swift with the Client ID and domain, run your app to verify that it is not producing any errors related to the SDK.
Login
Import the Auth0
module in the file where you want to present the login page.
import Auth0
Was this helpful?
Then, present the Universal Login page in the action of your Login button.
Auth0
.webAuth()
.useHTTPS() // Use a Universal Link callback URL on iOS 17.4+ / macOS 14.4+
.start { result in
switch result {
case .success(let credentials):
print("Obtained credentials: \(credentials)")
case .failure(let error):
print("Failed with: \(error)")
}
}
Was this helpful?
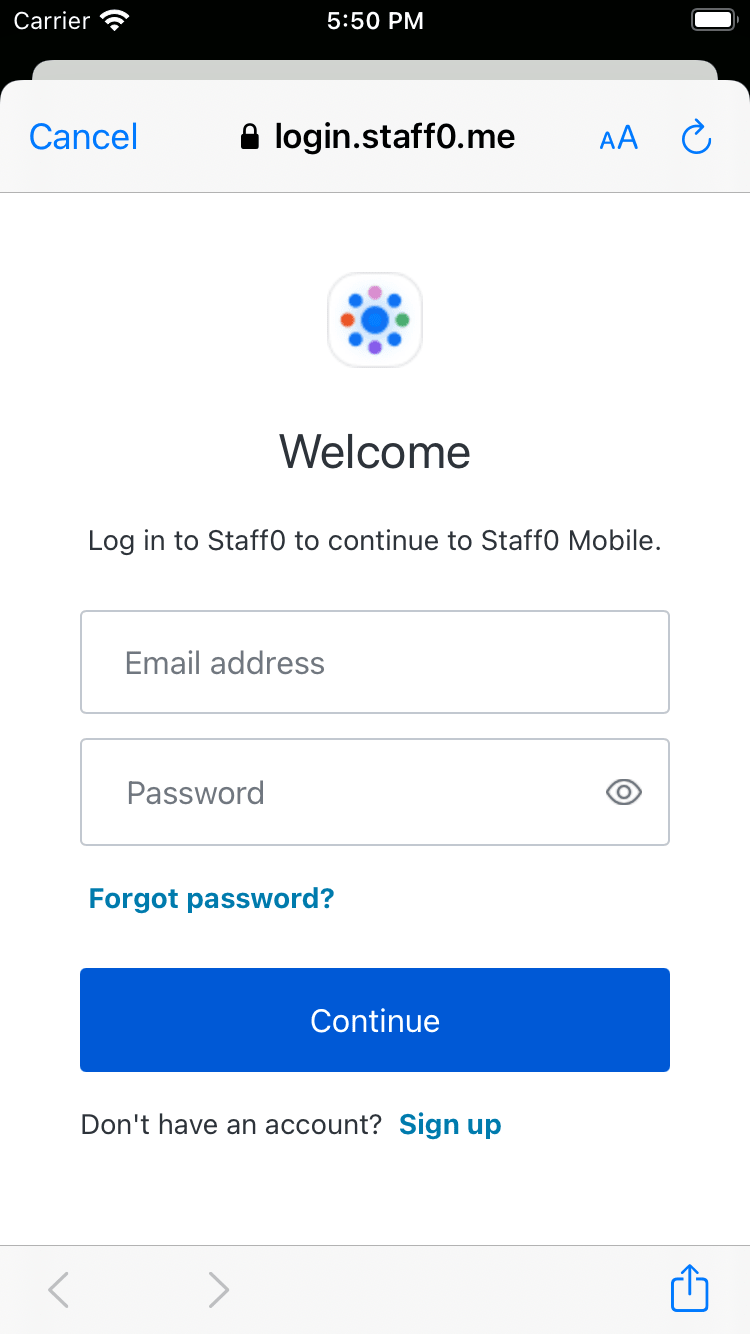
Checkpoint
Verify that pressing the Login button shows an alert box asking for consent and that choosing Continue opens the Universal Login page in a Safari modal. Verify that you can log in or sign up using a username and password or a social provider.
Once that is complete, verify that the Safari modal closes automatically.
Logout
Now that you can log in to your app, you need a way to log out. In the action of your Logout button, call the clearSession()
method to clear the Universal Login session cookie.
Auth0
.webAuth()
.useHTTPS() // Use a Universal Link logout URL on iOS 17.4+ / macOS 14.4+
.clearSession { result in
switch result {
case .success:
print("Logged out")
case .failure(let error):
print("Failed with: \(error)")
}
}
Was this helpful?
Checkpoint
Verify that pressing the Logout button shows an alert box asking for consent and that choosing Continue opens a page in a Safari modal. Verify that the Safari modal closes automatically soon after.
Access User Profile Information
The Credentials
instance you obtained after logging in includes an ID Token. The ID Token contains the profile information associated with the logged-in user, such as their email and profile picture. You can use these details to personalize the user interface of your app.
The Auth0.swift SDK includes a utility for decoding JWTs like the ID Token. Start by importing the JWTDecode
module in the file where you want to access the user profile information.
import JWTDecode
Was this helpful?
Then, use the decode(jwt:)
method to decode the ID Token and access the claims it contains.
guard let jwt = try? decode(jwt: credentials.idToken),
let name = jwt["name"].string,
let picture = jwt["picture"].string else { return }
print("Name: \(name)")
print("Picture URL: \(picture)")
Was this helpful?
Checkpoint
Verify that you can access the email
, picture
, or any other claim after you have logged in.
What's Next?
Check the SDK documentation to learn how to perform some common tasks, explore more advanced use cases, and discover all the available features: