Add Login to Your Flutter Application
Auth0 allows you to quickly add authentication and access user profile information in your application. This guide demonstrates how to integrate Auth0 with a Flutter application using the Auth0 Flutter SDK.
This quickstart assumes you already have a Flutter application up and running. If not, check out the Flutter "getting started" guides to get started with a simple app.
You should also be familiar with the Flutter command line tool.
When you signed up for Auth0, a new application was created for you, or you could have created a new one. You will need some details about that application to communicate with Auth0. You can get these details from the Application Settings section in the Auth0 dashboard.
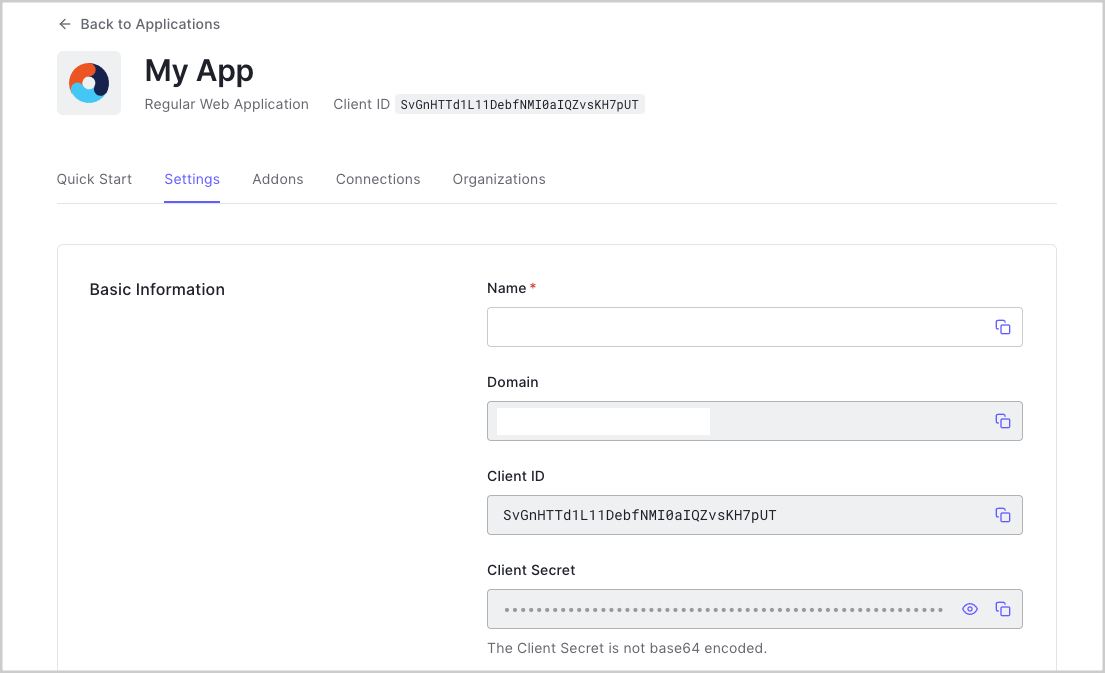
You need the following information:
Domain
Client ID
Configure Callback URLs
A callback URL is a URL in your application where Auth0 redirects the user after they have authenticated. The callback URL for your app must be added to the Allowed Callback URLs field in your Application Settings. If this field is not set, users will be unable to log in to the application and will get an error.
Configure Logout URLs
A logout URL is a URL in your application that Auth0 can return to after the user has been logged out of the authorization server. This is specified in the returnTo
query parameter. The logout URL for your app must be added to the Allowed Logout URLs field in your Application Settings. If this field is not set, users will be unable to log out from the application and will get an error.
Configure Allowed Web Origins
You need to add the URL for your app to the Allowed Web Origins field in your Application Settings. If you don't register your application URL here, the application will be unable to silently refresh the authentication tokens and your users will be logged out the next time they visit the application, or refresh the page.
Add the Auth0 Flutter SDK into the project:
flutter pub add auth0_flutter
Was this helpful?
Add the following script tag to your index.html
page:
<script src="https://cdn.auth0.com/js/auth0-spa-js/2.0/auth0-spa-js.production.js" defer></script>
Was this helpful?
Universal Login is the easiest way to set up authentication in your application. We recommend using it for the best experience, best security, and the fullest array of features.
Integrate Auth0 Universal Login in your Flutter Web app by using the Auth0Web
class. Redirect your users to the Auth0 Universal Login page using loginWithRedirect()
.
When a user logs in, they are redirected back to your application. You are then able to access the ID and access tokens for this user by calling onLoad
during startup and handling the credentials that are given to you:
auth0.onLoad().then((final credentials) => setState(() {
// Handle or store credentials here
_credentials = credentials;
}));
Was this helpful?
checkpoint.header
Add a button to your app that calls loginWithRedirect()
and logs the user into your app. Verify that you are redirected to Auth0 for authentication and then back to your application.
Verify that you can access credentials
as a result of calling onLoad
and that you're able to access the ID and access tokens.
To log users out, redirect them to the Auth0 logout endpoint to clear their login session by calling the Auth0 Flutter SDK logout()
. Read more about logging out of Auth0.
checkpoint.header
Add a button to your app that calls logout()
and logs the user out of your application. When you select it, verify that your Flutter app redirects you to the logout endpoint and back again. You should not be logged in to your application.
The user profile automatically retrieves user profile properties for you when the page loads, and can be accessed and stored by calling onLoad
during application startup. The returned object from onLoad
contains a user
property with all the user profile properties. This is internally populated by decoding the ID token.
checkpoint.header
Log in and inspect the user
property on the result. Verify the current user's profile information, such as email
or name
.
Next Steps
Excellent work! If you made it this far, you should now have login, logout, and user profile information running in your application.
This concludes our quickstart tutorial, but there is so much more to explore. To learn more about what you can do with Auth0, check out:
- Auth0 Dashboard - Learn how to configure and manage your Auth0 tenant and applications
- auth0-flutter SDK - Explore the SDK used in this tutorial more fully
- Auth0 Marketplace - Discover integrations you can enable to extend Auth0’s functionality
Sign up for an or to your existing account to integrate directly with your own tenant.