At Auth0, we believe that extensibility is one of our most important product features.
Identity for one customer is not always the same thing for the next. Every customer has specific needs, and a vast majority want to customize our core product to solve their requirements. We are committed to continually looking for ways to enhance our product capability to answer this call.
Answering the Call
Because every project has a unique use case, we’ve learned over the years that sometimes, just simply building features into our core product is not the right approach. Instead, we are continuously brainstorming new tools that will allow you to customize your identity solution as needed.
Auth0 Rules was our first publicly available tool that looked to answer extensibility needs. Rules are serverless code blocks that can be customized to extend Auth0's core capabilities.
They execute after authentication but before identity tokens are issued. Our documentation offers a great list of Rule templates that make it easy to tackle common scenarios out of the box. They can be chained together for modular coding and turned off individually.
Rules are powerful and solve many authentication use cases. We learned through iteration that customers want customization capabilities beyond the login flow.
In response to this learning, we introduced Auth0 Hooks.
Hooks allow custom behavior using a similar custom code setup as Rules. However, they can be executed against several extensibility points. This includes the client credentials exchange as well as pre and post-registration.
Through several learning iterations, we discovered that even though Hooks can run on more extensibility points than Rules, customers want even more capability from our extensibility platform.
That is why we are excited to announce our next-gen extensibility platform — Auth0 Actions!
What Are Auth0 Actions?
Actions are just another tool in your identity solution toolbelt to meet your application needs.
Actions are functional services that fire during specific events across multiple identity flows.
This behavior works as a trigger system. The triggers that kick off an Action are extensibility points such as post-login or client credential exchange. The Actions platform is designed for Auth0 to continuously add additional triggers as our core product develops.
Action Features
The Actions platform comes with:
✋ Drag N Drop Functionality — The flow editor lets you visually build custom workflows with drag and drop Action blocks for complete control.
💻 Monaco Code Editor with Quick-hints — Designed with developers in mind, you can easily write JavaScript functions with validation, intelligent code completion, and type hints.
You can also securely store and use secrets within your custom functions. Once they are saved, they cannot be read in plain text in the code again.
We have also improved the functionality of your code by allowing access to the entire library of NPM packages and modules.
🏢 Serverless (Function as a Service, FaaS) Environment — We host your custom Action functions and process them when desired. The functions are stored and run on our own infrastructure. This improves overall performance and security for our customers.
💾 Version Control — Everything you love about Git and version control is now available for your custom Actions. You can store a history of individual Action changes and the power to revert back to previous versions as needed.
📁 Pre-Production Testing — Outside of Actions, custom extensible code cannot be tested unless rolled to your production environment. We are happy to announce that with Actions, your personal Actions can be drafted, reviewed, and tested before deploying into production
🐛 Debugging — Nothing is worse than getting an error and not having the ability to track down the problem. Actions provide the ability to determine causality by tracking state changes.
Note: If you are a pro user wondering how this affects Rules and Hooks, don’t worry, as Actions will function separately.
We want to win you over with Actions!
Create Your First Auth0 Action
Let’s walk through creating your first Action! We'll create an action that will limit access to your application to only Monday through Friday.
Login to your Auth0 account. If you don't have one, you can get it for free. In the left sidebar, there's a section titled “Actions.” Click on that. You’ll see two subcategories, “Flows” and “Library.” Click Flows.
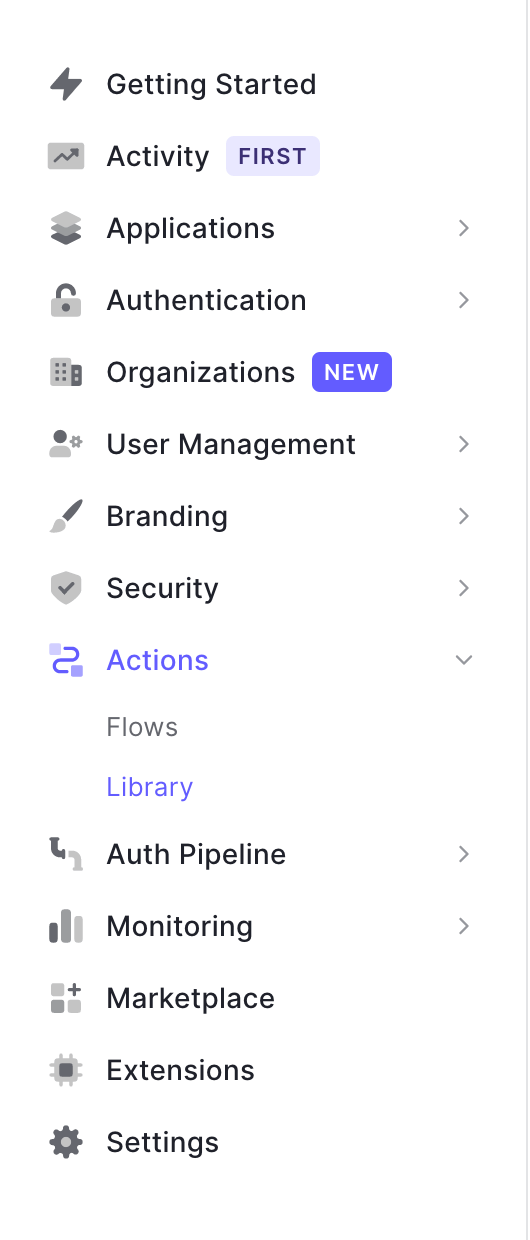
Flows represent a high-level operation within Auth0. The current flows you can add Actions to are “Login,” “Machine to Machine," "Pre User Registration," "Post User Registration," Post Change Password," and "Send Phone Message." For this example, click Login.
Once you click on Login, you should see the following page:
This is the flow editor. It shows the entire authentication flow for your logging in. You get drag and drop functionality to customize the flow. If your solution has any Rules already set up, you will see them in this view. Any Rules that are defined will be triggered first, and then they will flow into your Actions.
In the top right corner of this view there is a small question mark icon. Clicking this will show a pop-over walkthrough of this view.
Click on the + option on the right side, then click Build Custom on the pop-in menu.
On the "Create Action" menu, name this Action “Weekdays Only” and click Create.
Now, we're in the new and improved Actions code editor, where we can customize our new "Weekdays Only" action.
On the upper right side, we have buttons for "Version history," "Save Draft," and "Deploy."
Unlike with Rules and Hooks, the code editor holistically supports:
- Testing
- Secret management
- Virtually any NPM module
- Linting
- Quick hints
Also, async
/ await
is set up by default, so you do not need to use callbacks.
In order to see the custom hint functionality, press CMD
+ space
(CTRL
+ space
on Windows). Also, by simply hovering over objects such as event
and api,
you will see their documentation in a pop-up.
Notice on the left side of the editor is a couple of icons. The play icon is how we can test our Action before deploying it; more on that in a little bit.
The key icon is how we can add and use secrets in our Actions. For example, when working with APIs, such as the Slack API, we want to make sure we do not expose any API keys accidentally. This is where we can store it once, keep it secure, and reference it in our Actions editor.
Finally, the last box icon is where you add NPM modules in our Actions.
Write custom code for Actions
Let’s write the code for our Action. We will write an Action that restricts access to the application only during weekdays after logging in.
The code editor has the async JavaScript method onExecutePostLogin
pre-populated. Any code we write in this function will run after a user logs in.
By default, Actions apply to all applications in your Auth0 tenant.
First, we'll create a variable called dayOfWeek
and set it to the return value of new Day.getDay()
.
The getDay()
method returns the day of the week according to local time represented by a number (0 = Sunday, 1 = Monday, etc.)
exports.onExecutePostLogin = async (event, api) => {
const dayOfWeek = new Date().getDay();
};
Let's create an if
statement to check which day of the week our user is logging in on. We'll check if our dayOfWeek
variable is 0 (Sunday) or 6 (Saturday)
If dayOfWeek
is equal to 0 or 6, we'll deny the user access with the api
object with api.access.deny
and pass in the string "This app is only available during the week."
Learn more about the API object by reading the Auh0 Actions API object docs
exports.onExecutePostLogin = async (event, api) => {
const dayOfWeek = new Date().getDay();
if (dayOfWeek === 0 || dayOfWeek === 6) {
api.access.deny("This app is only available during the week.");
}
};
If you're logging in during the week and want to see this message, change the number in the
if
statement to match what day of the week it is.
Test Auth0 Actions
We can test it before pushing it to production to ensure this Actions code works. We do this by pressing the play icon on the left sidebar and clicking "Run" at the bottom. This will run our code and display the output on the bottom of the editor. We also can test different scenarios by updating the test data.
Click Save draft. We can also revert this action to a previously saved or deployed version by pressing the "Version Control" button on the top of this page. We would see all saved versions with a push-button revert process.
Now that we’ve successfully tested our Action, we can deploy it by clicking on Deploy. If an action is not deployed, it does not appear in our flow Action list.
Add Action to login flow
Now, let’s actually add the Action just created to our flow. Click on the “Flows” tab on the left side navigation and click "Login."
This will take us back to our flow builder, and our "Weekdays Only" Action is now available. Drag the Action and drop it between "Start" and "Complete" in the login flow.
Once we’ve added our Action to the Flow, click Save, then Apply.
Now let’s try it out.
You can give this a quick test drive without running your full app, by going to the right side menu in the Auth0 dashboard and clicking Getting Started. On the Getting Started page, click Try it out in the Try your Login box section.
After you click Try it out, you'll be brought to a login screen where you can enter an email and password. Proceed to log in. Once the credentials are entered, you'll see a Bummer! Something failed page with the error "access_denied"
and an error description of "This app is only available during the week."
And that's all!
With Auth0 Actions, we were able to:
- Quickly create a reusable custom Action with a built-in user-friendly code editor
- Test, save, and rollback to different versions of the flow
- Attach it to a trigger
Go ahead and add some on your own and let us know what you think in the comments below!
There are some limits to using Auth0 Actions. To view the most up to date limitations, head over to the Actions docs.
What's Coming?
Actions will be an iterative platform that we will continually improve upon. Our vision for Actions includes expanding the number of triggers (extensibility points) you can use to customize Auth0 and introducing new ways to reuse code, so you don’t have to keep writing and maintaining large amounts of custom code.
We'd love to hear what you think about Actions. Be sure to comment let us know of any future integrations you'd like to see.
This is just the beginning of Auth0 Actions, and we can't wait to see where we can take it with your feedback!