AngularJS Authentication, the Easy Way
Add token authentication to your Angular 1.x or Angular 2 app in minutes with ready-to-go tools.
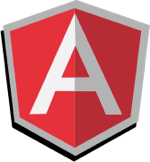
What Is AngularJS?
AngularJS is one of the world’s most popular JavaScript frameworks for creating Single Page Applications. Developed by Google, Angular provides a host of tools that make it easy to wire up the various pieces that are required for a SPA. Things like two-way databinding, HTTP requests, templating, and routing are made simple with the AngularJS core library and third-party libraries contributed by the community.
As with other SPA frameworks, Angular is only concerned with the front end and is agnostic about the server side. This decoupling, along with the client-side architecture that Angular provides, ultimately allows for greater flexibility as applications grow.
AngularJS Authentication from scratch can be a hassle, but it doesn’t need to be. Keep reading below to find out how Auth0 makes it easy to add AngularJS authentication.
Tokens Work Best for Single Page Apps
AngularJS applications work differently than traditional round-trip applications. Like other Single Page App (SPA) frameworks, AngularJS apps typically rely on data APIs which are accessed by sending XHR requests from the client to the server.
Adding user authentication to AngularJS apps is also different. Traditional applications use session-based authentication which works by keeping the user’s authentication state saved in memory on the server, but this doesn’t work so well for SPAs.
Tokens offer a better way to achieve AngularJS Authentication. Several different types of authentication tokens can work, but JSON Web Tokens are the best solution.
JSON Web Tokens
JSON Web Token (JWT) is an open standard (RFC 7519) that defines a compact and self-contained way for securely transmitting information between parties as a JSON object. There are many reasons that JWT authentication is preferable:
Compact and self-contained: all data needed for authentication exists in the token. It can be transmitted quickly because of its small size.
Digitally signed: tokens are verified against a secret key on the server. They are secure because the content of the JWT can’t be tampered with unless the secret key is known.
Simple: JWTs are conceptually straight-forward and have low overhead. Since they provide a stateless means for authentication, they can be used across multiple servers and domains without running into CORS issues.
AngularJS Authentication with Auth0 - How It Works
With Auth0, your AngularJS app only needs to talk to our API when the user logs in. All other API calls go directly to your server as they normally would.
Using either our Lock Widget or your own custom login screen, your users send their credentials to our API to be authenticated. Upon success, a JWT is returned and saved in their browser’s local storage.
auth.signin({}, function(profile, token) {
// Success callback
store.set('profile', profile);
store.set('token', token);
$location.path('/');
});
API endpoints that you wish to secure are protected with middleware that requires a valid JWT to be sent in HTTP requests. The user’s JWT is sent as an Authorization header and is verified against your secret key. A jwtInterceptor is configured to send the user’s JWT on all requests.
jwtInterceptorProvider.tokenGetter = ['store', function(store) {
// Return the saved token
return store.get('token');
}];
$httpProvider.interceptors.push('jwtInterceptor');
Lock - The Login Box Done Right
Our Lock widget is a beautifully designed, all-in-one, embeddable login box for your AngularJS apps. It provides sign up, sign in, password reset, and other features that are ready to go. The widget’s styles can be easily customized to line up with your brand, and you can use one of our pre-made themes to help.
Angular 2 Authentication Support
Angular 2 has yet to be officially released and is not recommended for production, but you can still use Auth0 in your Angular 2 apps. Our angular2-jwt helper library makes it easy to send authenticated HTTP requests to your server and to handle routing based on the user’s authentication state.
Sign up for free
Start building today and secure your apps with the Auth0 identity platform today.
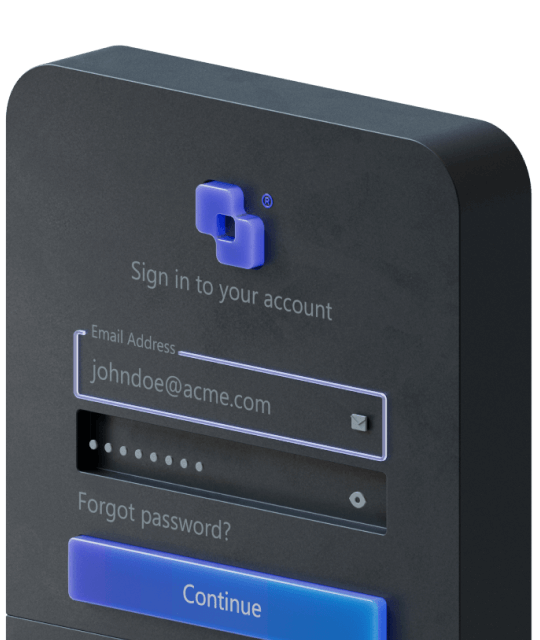