Pre-user Registration Trigger
The Pre-user Registration trigger runs before a user is added to a Database or Passwordless Connection.
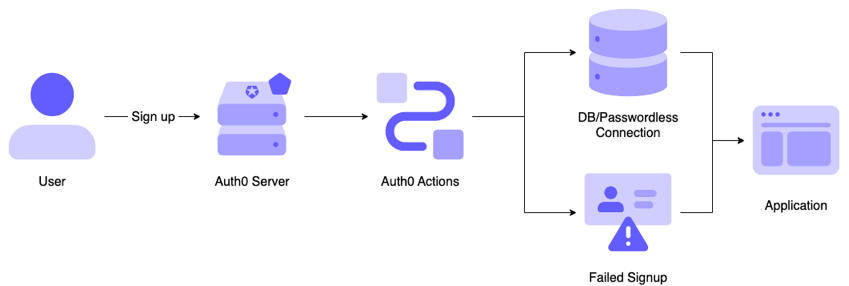
Actions in this flow are blocking (synchronous), which means they execute as part of a trigger's process and will prevent the rest of the Auth0 pipeline from running until the Action is complete.
Triggers
Pre-user Registration
The pre-user-registration
triggers runs when a user attempts to register through a Database or Passwordless connection. This trigger can be used to add metadata to the user profile before it is created or to deny a registration with custom logic.
References
Event object: Provides contextual information about the request to register a new user.
API object: Provides methods for changing the behavior of the flow.
Common use cases
Deny registration by location
A pre-user registration Action can be used to prevent a user from signing up.
/**
* @param {Event} event - Details about registration event.
* @param {PreUserRegistrationAPI} api
*/
exports.onExecutePreUserRegistration = async (event, api) => {
if (event.request.geoip.continentCode === "NA") {
// localize the error message
const LOCALIZED_MESSAGES = {
en: 'You are not allowed to register.',
es: 'No tienes permitido registrarte.'
};
const userMessage = LOCALIZED_MESSAGES[event.request.language] || LOCALIZED_MESSAGES['en'];
api.access.deny('no_signups_from_north_america', userMessage);
}
};
Was this helpful?
Set metadata in the user profile
A pre-user registration Action can be used to add metadata to the user profile before it is created.
/**
* @param {Event} event - Details about registration event.
* @param {PreUserRegistrationAPI} api
*/
exports.onExecutePreUserRegistration = async (event, api) => {
api.user.setUserMetadata("screen_name", "username");
};
Was this helpful?
Store a user ID from another system in the user profile
A pre-user registration Action can be used to store a user ID from another system in the user profile.
const axios = require('axios');
const REQUEST_TIMEOUT = 2000; // Example timeout
/**
* Handler that will be called during the execution of a PreUserRegistration flow.
*
* @param {Event} event - Details about the context and user that is attempting to register.
* @param {PreUserRegistrationAPI} api - Interface whose methods can be used to change the behavior of the signup.
*/
exports.onExecutePreUserRegistration = async (event, api) => {
try {
// Set a secret USER_SERVICE_URL = 'https://yourservice.com'
const remoteUser = await axios.get(event.secrets.USER_SERVICE_URL, {
timeout: REQUEST_TIMEOUT,
params: {
email: event.user.email
}
});
if (remoteUser) {
api.user.setAppMetadata('my-api-user-id', remoteUser.id);
}
} catch (err) {
api.validation.error('custom_error', 'Custom Error');
}
};
Was this helpful?