Test Actions
Depending on where you are in your implementation, you have several different options available to test and debug Auth0 Actions.
We recommend that you test individual actions from the Auth0 Dashboard as you implement them. Once you are ready, you should verify that the end-to-end flow works as expected using tenant logs through the Auth0 Dashboard.
Test individual Actions
You can test individual Actions using the Actions Code Editor. The editor's test capability simulates a call to the Action using a sample payload based on the flow with which the Action is associated. To test an individual Action:
Navigate to Auth0 Dashboard > Actions, and choose the Flow and Action that you would like to edit.
Locate the Actions Code Editor, and select Test (the play icon) from its sidebar. Edit the payload in the Test sidebar to analyze the outcome of different payloads.
Select Run.
The test results show the steps that the Action took, console output, any errors that occurred, and useful statistics about the execution of the Action.
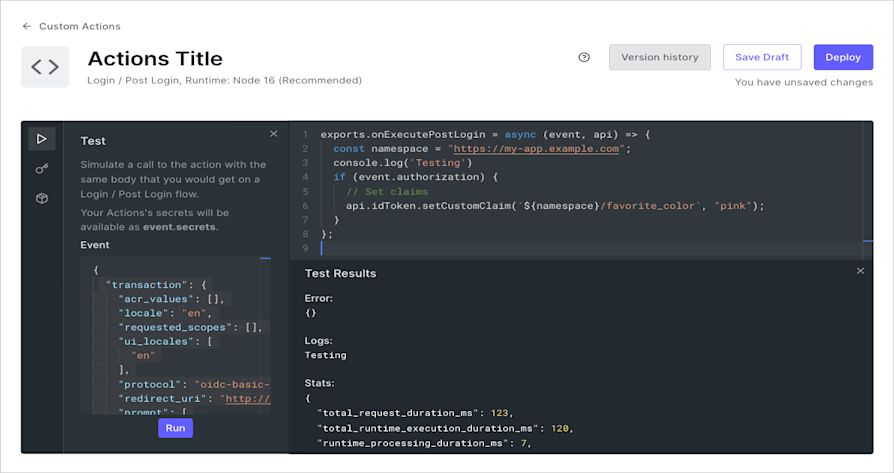
Debug deployed Actions
Now that you have tested your Action, it’s time to deploy it and observe its execution. Assuming you have deployed your Action and added it to a flow (to learn how to attach Actions to Flows, read the "Attach the Action to a flow" section in Write Your First Action), you can debug your live Action in a few steps.
Verify end-to-end Login Flow
For a post-login
Action, you can verify the end-to-end-login flow by executing a login attempt for your tenant:
Navigate to Auth0 Dashboard > Authentication > Authentication Profile, and select Try. A window containing a sample login will open.
Proceed through the login flow. The login flow will execute any configured Actions.
Once complete, you will be redirected to a page that either lists the user profile attributes that your applications will receive or shows an error message explaining what went wrong.
Analyze tenant logs
Whenever a trigger executes that has an associated Action bound to its flow, your tenant logs will include Action execution details.
In the tenant logs list, you can see logs associated with Actions and choose to view them. In the example below, the log type of Success Login
is associated with an Action on the post-login
trigger of this tenant.
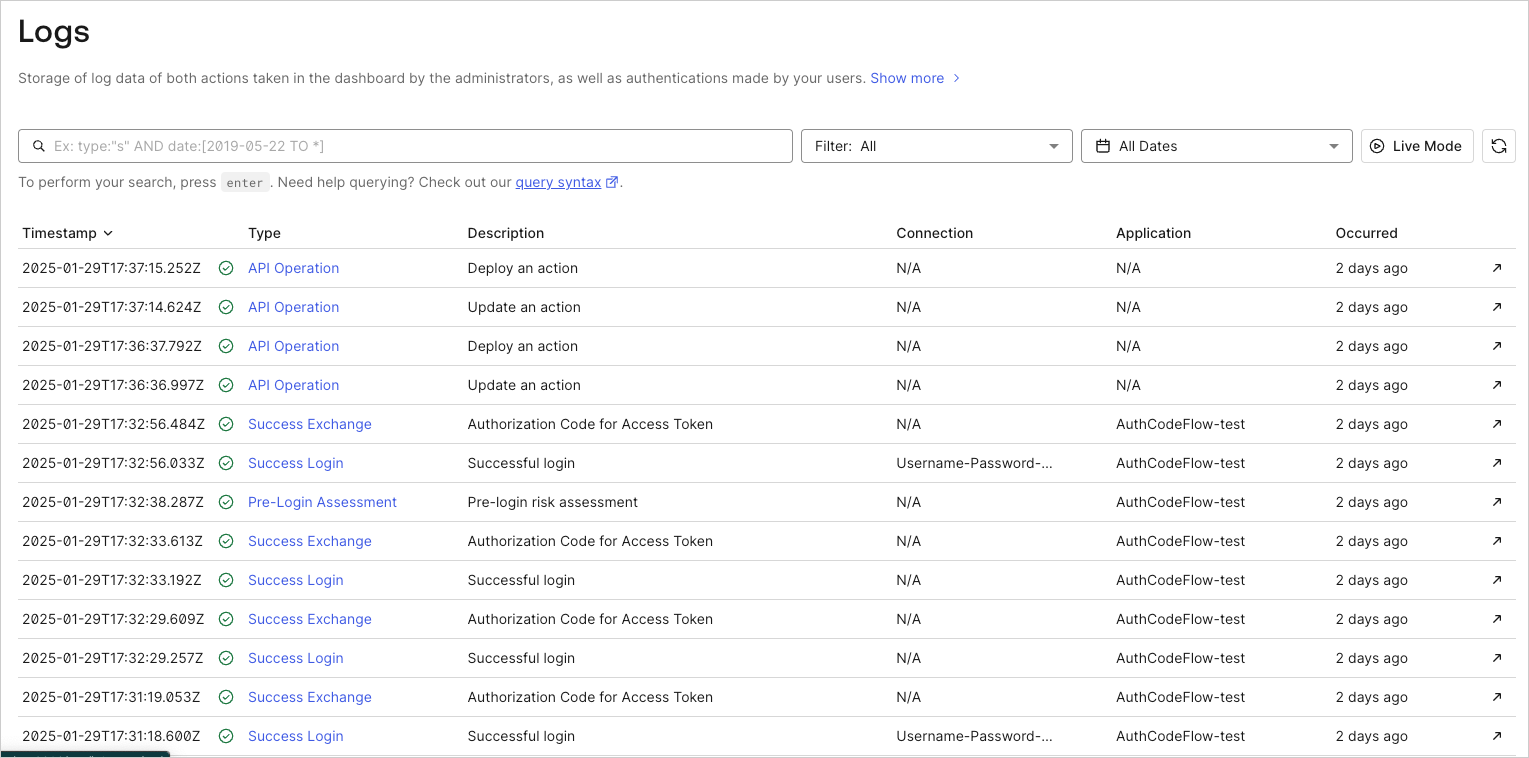
Some examples of log types associated with Actions include:
Name | Trigger(s) |
---|---|
Success Login | post-login |
Failed Login | post-login |
Success Exchange | post-login |
Failed Exchange | post-login |
Success Change Password | post-change-password |
Failed Change Password | post-change-password |
Successful Signup | pre-user-registration |
Failed Signup | pre-user-registration |
Success Exchange | credentials-exchange |
Failed Exchange | credentials-exchange |
To view detailed Action execution information, select the log that is associated with an Action execution, then select the Action Details view. In Action Details, you can see execution statistics, console output, and any errors that may have occurred.
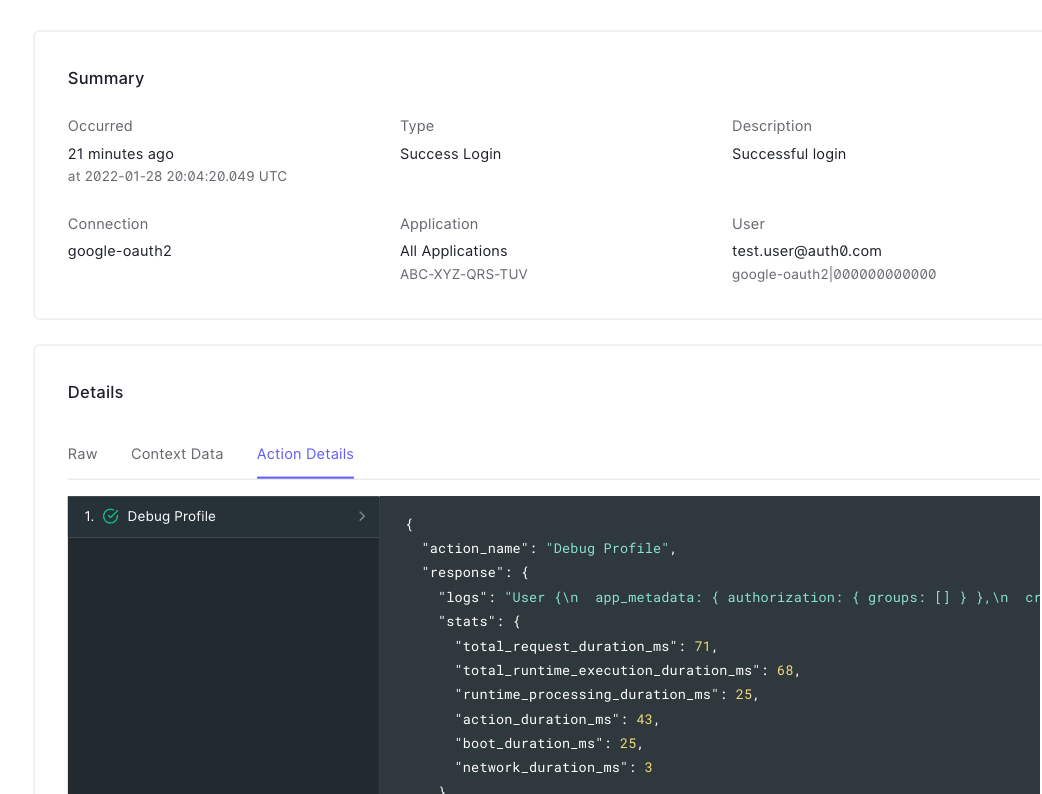
Unit test Actions
You can unit test your Auth0 Actions by mocking the event and api passed into your Action function. Your Actions can live in version control or local directory, and you can use a tool like Jest to automatically test your Actions. In the following example, we use the Login / Post Login trigger and Jest to mock and test.
Action code:
// action.js
exports.onExecutePostLogin = async (event, api) => {
const namespace = "https://my-app.example.com";
if (event.authorization) {
api.idToken.setCustomClaim(`${namespace}/favorite_color`, "pink");
}
};
Was this helpful?
Test file code:
// action.spec.js
const { onExecutePostLogin } = require("./action.js");
test("sets favorite_color ID token claim to pink", async () => {
const namespace = "https://my-app.example.com";
const mockFunction = jest.fn();
const mockApi = { idToken: { setCustomClaim: mockFunction } };
const mockEvent = {authorization: {}};
await onExecutePostLogin(mockEvent, mockApi);
expect(mockFunction).toBeCalledWith(
`${namespace}/favorite_color`,
"pink"
);
});
Was this helpful?
Best practices
When building Actions on Auth0, we recommend that you create a dedicated Auth0 tenant per environment. Doing so allows you to isolate your production user base from your other environments, as well as configure different administrators per environment (for example, engineers might not have access to production configuration).