Add Sign In with Google to Native Android Apps
Sign in with Google allows users to authenticate with an active Google Account, providing users with a seamless login experience for your application. You can implement this feature for your native Android applications using the Credential Manager for Android with Auth0.
Review the sections below to learn more about the steps and methods required for configuring Sign in with Google.
How it works
This feature uses Android's Credential Manager to facilitate Sign in with Google in your Auth0-protected Android application. The steps below demonstrate the general user workflow for Sign in with Google.
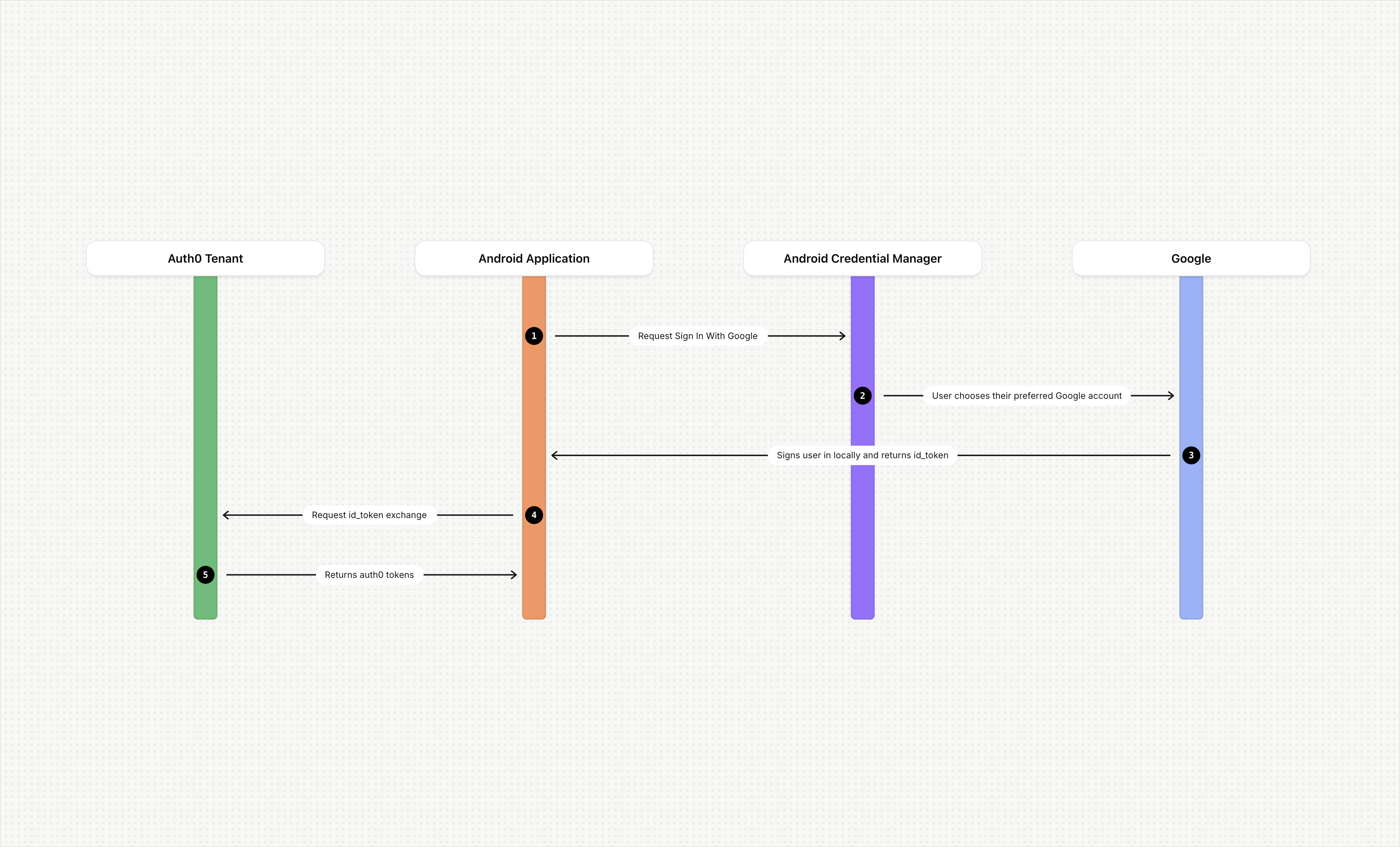
A user opens your Android application and chooses to log in with Google.
Your Android application uses the Credential Manager to request Sign in with Google.
On the account selection prompt, the user chooses their preferred Google account.
Google signs the user in locally and handles all authentication.
The user completes sign-in with no additional interaction required.
Google returns an
id_token
to your Android application.Your Android application sends the
id_token
to your Auth0 tenant for validation. Auth0 validates theclient_id
from theid_token
against theclient_id
of the Google social connection configured in your tenant.The Auth0 server returns an
access_token
to your Android application.
Before you begin
Before you begin configuring Sign in with Google, ensure the following are true:
A Google social connection has been set up within your Auth0 tenant.
Sign in with Google has been added to your Android application using Android's Credential Manager.
Configuring Sign in with Google for Android applications
Implementing Sign in with Google involves three primary steps:
Creating credentials in Google Cloud Console.
Configuring application details in Auth0.
Updating code in your Android application.
The sections below provide technical details for each of these steps.
Create Credentials in the Google Cloud Console
To get started, you must first configure the following items in your Google Cloud Console:
Create an OAuth 2.0 credential with the type set to
Android
. This is referred to asclient_id_native
.Add the SHA1 hash for the native application to the Android Client from Step 1.
Google currently only supports SHA1.
Create an additional OAuth client for web (
client_id_web
).In some scenarios, you may have already configured this item for your social connections that support web-based Sign in with Google.
Configure Auth0
The Sign in with Google flow leverages the OAuth 2.0 Token Exchange, which occurs between Auth0 and your Android application.
After creating your credentials in the Google Cloud Console, you can enable Sign in with Google using the Credential Manager for Android. To do so, update your application through either the Auth0 Dashboard or the Management API.
To update the application through the Auth0 Dashboard, follow the steps below:
Navigate to Applications > Applications and choose your native Android application.
On the Settings tab, expand the Advanced Settings section.
Select the Device Settings tab and activate the Enable Sign in with Google (Android 4.4+) using Credentials Manager setting.
For new applications: On the Device Settings tab, complete the fields in the Android section, including App Package Name. For more information, review Enable Android App Links Support.
Select Save Changes.
To update your application through the Management API, call the Update a client endpoint as follows:
PATCH /api/v2/clients/{id}
{
"native_social_login": {
"google": {
"enabled": true
}
},
"mobile": {
"android": {
// Update this to reflect the bundle identifier of your App
"app_package_name": "com.yoursite.yourapp"
}
}
}
Was this helpful?
Update code in the Android application
The following example can complement the web flow used for non-Google authentications, such as Microsoft, username and password, or enterprise federations.
auth0Client.loginWithNativeSocialToken(
googleCredential.idToken.toString(),
"http://auth0.com/oauth/token-type/google-id-token")
.validateClaims()
.setScope("openid profile email")
.start(object: Callback < Credentials, AuthenticationException > {
override fun onFailure(error: AuthenticationException) {
showSnackBar("Failure a0: $error")
}
override fun onSuccess(auth0Creds: Credentials) {
// Logic to Handle credentials
}
})
Was this helpful?
Obtaining the Google googleCredential
requires the native application code to invoke the Google library. For details, refer to Google's Credential Manager documentation.
// Generate a nonce for token replay protection
val randomNonce = UUID.randomUUID().toString()
// Prepare a Sign in request
val googleIdOption: GetGoogleIdOption = GetGoogleIdOption.Builder()
// This must match the Client ID Configured in the auth0 dashboard
.setServerClientId(
getString(R.string.com_google_client_id)
)
.setNonce(randomNonce)
.build()
val request: GetCredentialRequest = GetCredentialRequest.Builder()
.addCredentialOption(googleIdOption)
.build()
// To Prompt
val credMan = CredentialManager.create(this@MainActivity.baseContext);
val result = credMan.getCredential(
request = request,
context = this@MainActivity.baseContext,
)
// Handle outcome
val creds = result.credential
when (creds) {
is CustomCredential -> {
if (creds.type ==
GoogleIdTokenCredential.TYPE_GOOGLE_ID_TOKEN_CREDENTIAL
) {
try {
val googleCredentials = GoogleIdTokenCredential
.createFrom(creds.data)
// Rest of the code
Was this helpful?
Additional authentication scenarios
While the current Google implementation does not offer clear guidance for enterprise federation and additional multi-factor authentication (MFA) challenges, you can accommodate these capabilities by transitioning to the web experience.
Enterprise federation
Auth0 supports enterprise federation, which allows you to connect external enterprise identity providers (such as Okta Workforce, ADFS, or other OIDC-compatible systems) to your Auth0 tenant so that users can then authenticate with their existing corporate credentials.
If you require the use of enterprise federation, redirecting the user to the web-based flow is recommended, instead of relying on the id_token
as demonstrated in previous examples.
To do so, decode the Google JWT token and pass the email
provided in the ID token as a login_hint
. This prevents the end user from having to re-enter their email address in the web flow.
if (error.isAccessDenied && reason == "Enterprise Domain") {
WebAuthProvider.login(account)
.withParameters(mapOf("login_hint" to email))
.start(...)
}
Was this helpful?
MFA considerations during token exchange
Auth0 returns an error to the application during token exchange when the Google OAuth connection policy requires multi-factor authentication. This error is included in the response to the application.
When the above error occurs, your application can use WebAuth with the enriched context available in the Google id_token
. In this scenario, the end user will only see the MFA screen.
if (error.isMultifactorRequired) {
WebAuthProvider.login(account)
.withParameters(mapOf(
"login_hint" to email,
"connection" to "google-oauth-2"
))
.start(...)
}
Was this helpful?