Guardian.swift iOS SDK
要件
Guardianを使用するにはiOS 10+とSwift 4.1が必要です。
このSDKを使用するには、テナントのGuardianサービスに独自のプッシュ通知資格情報を構成する必要があります。そうしないと、プッシュ通知が届きません。詳細については、「MFAにプッシュ通知を構成する」を参照してください。
Guardian iOS SDKをインストールする
CocoaPods
Guardian.swiftはCocoaPodsから入手可能です。インストールするには、以下の行をPodfileに追加します。
pod 'Guardian', '~> 1.1.0'
Was this helpful?
Carthage
以下の行をCartfileに追加します。
github "auth0/Guardian.swift" ~> 1.1.0
Was this helpful?
Guardianプッシュ通知を有効にする
[Dashboard] > [Security(セキュリティ)] > [Multi-factor Auth(多要素認証)]に進みます。
[Push Notification(プッシュ通知)]を切り替えて有効にします。
Usage(使用)
Guardian
はSDKの中核です。SDKを使用するには、ライブラリをインポートします。
import Guardian
Was this helpful?
テナントのドメインを設定します。または、テナントにカスタムドメインを構成している場合、それを使用します。
let domain = "<tenant>.<region>.auth0.com"
Was this helpful?
登録
登録は、第二要素とAuth0アカウント間のリンクです。アカウントが登録されると、本人確認に必要な第二要素を提供するためにアカウントが必要になります。アプリがまだプッシュ通知を使用していない場合、またはプッシュ通知に慣れていない場合、「Appleプッシュ通知サービスの概要」を参照してください。
登録には、テナントのドメイン以外に以下の情報が必要です。
変数 | 説明 |
---|---|
Enrollment URI(登録URI) | Guardian WebウィジェットからスキャンしたQRコード、またはメールかSMS経由で送られてきた登録チケットにエンコードされた値。 |
APNS Token(APNSトークン) | デバイスのApple APNSトークン。64バイト(16進数形式)を含む文字列で指定する必要があります。 |
Key Pair(鍵ペア) | Auth0 GuardianでIDをアサ―トするのに使用するRSA(秘密/公開)鍵ペア。 |
情報を入手した後、デバイスを登録できます。
Guardian
.enroll(forDomain: "{yourTenantDomain}",
usingUri: "{enrollmentUri}",
notificationToken: "{apnsToken}",
signingKey: signingKey,
verificationKey: verificationKey
)
.start { result in
switch result {
case .success(let enrolledDevice):
// success, we have the enrollment device data available
case .failure(let cause):
// something failed, check cause to see what went wrong
}
}
Was this helpful?
成功すると、登録情報を取得できます。その登録情報は、アプリケーションにセキュアに保存する必要があります。この情報には、登録識別子と、登録を更新または削除するためにデバイスに関連付けられたGuardian APIのトークンが含まれます。
署名キーと検証キー
Guardian.swiftは、署名鍵を生成するための便利なクラスを提供しています。
let signingKey = try DataRSAPrivateKey.new()
Was this helpful?
このキーはメモリ内にしか存在しません、データ
表現を取得し、暗号化されたSQLiteDBなどにセキュアに保存できます。
// Store data
let data = signingKey.data
// perform the storage
// Load from Storage
let loadedKey = try DataRSAPrivateKey(data: data)
Was this helpful?
しかし、iOSキーチェーンに保存したいだけなら、以下の手順で可能です。
let signingKey = try KeychainRSAPrivateKey.new(with: "com.myapp.mytag")
Was this helpful?
上記の例では、キーを作成し、与えられたタグの下に自動的に保存します。それを取得したい場合、タグを使用できます。
let signingKey = try KeychainRSAPrivateKey(tag: "com.myapp.mytag")
Was this helpful?
検証キーは、SigningKey
から取得できます。以下の例を挙げます。
let verificationKey = try signingKey.verificationKey()
Was this helpful?
ログイン要求を許可する
登録を行うと、MFAで本人確認が必要になるたびにプッシュ通知が届きます。Guardianは、APNから受信したデータを解析し、使用可能なNotification
インスタンスを返すメソッドを提供します。
if let notification = Guardian.notification(from: notificationPayload) {
// we have received a Guardian push notification
}
Was this helpful?
通知インスタンスを取得すると、allow
メソッドを使用して簡単に認証要求を許可できます。以前に取得した登録済みデバイスからの情報も必要です。複数の登録がある場合、必ず通知と同じid
(enrollmentId
プロパティ)のものを見つける必要があります。
情報があれば、device
パラメーターはプロトコルAuthenticatedDevice
を実装しているものとなります。
struct Authenticator: Guardian.AuthenticationDevice {
let signingKey: SigningKey
let localIdentifier: String
}
Was this helpful?
ローカル識別子はデバイスのローカルIDであり、デフォルトでは登録UIDevice.current.identifierForVendor
にあります。次に、以下を呼び出します。
Guardian
.authentication(forDomain: "{yourTenantDomain}", device: device)
.allow(notification: notification)
.start { result in
switch result {
case .success:
// the auth request was successfuly allowed
case .failure(let cause):
// something failed, check cause to see what went wrong
}
}
Was this helpful?
ログイン要求を拒否する
認証要求を拒否するには、代わりにreject
を呼び出します。オプションで拒否の理由を送ることもできます。拒否の理由はGuardianログに表示されます。
Guardian
.authentication(forDomain: "{yourTenantDomain}", device: device)
.reject(notification: notification)
// or reject(notification: notification, withReason: "hacked")
.start { result in
switch result {
case .success:
// the auth request was successfuly rejected
case .failure(let cause):
// something failed, check cause to see what went wrong
}
}
Was this helpful?
登録解除する
登録を削除したい場合、たとえばMFAを無効にしたい場合は、以下の要求を行います。
Guardian
.api(forDomain: "{yourTenantDomain}")
.device(forEnrollmentId: "{userEnrollmentId}", token: "{enrollmentDeviceToken}")
.delete()
.start { result in
switch result {
case .success:
// success, the enrollment was deleted
case .failure(let cause):
// something failed, check cause to see what went wrong
}
}
Was this helpful?
モバイル専用OTP登録をセットアップする
Auth0 DashboardまたはManagement APIを使用して、MFA要素としてワンタイムパスワード(OTP)を有効にすることができます。このオプションにはQRコードは必要なく、ユーザーが手動で登録できます。
ユーザーを登録に招待するには、Auth0 Dashboard > [User Management(ユーザー管理)] > [Users(ユーザー)]に進み、ユーザーを選択します。その後、[Details(詳細)]タブにアクセスし、[Multi-Factor Authentication(多要素認証)]セクションを使用して登録招待を送信します。
リソースを接続する
リソースは、Auth0 DashboardまたはGuardian SDKを使って接続できます。
Auth0 Dashboardの使用
Auth0ログインプロンプトにアクセスし、提供されたコード、または別のソースから取得した同様のbase32エンコードキーをコピーします。次のステップでは、このコードを認証アプリケーションに入力します。
コピーしたコードをGuardianなどの認証アプリケーションに追加します。
SDKを使用する
Guardianライブラリをインポートします。
import Guardian
Was this helpful?
/コードジェネレーターを作成します。
let codeGenerator = try Guardian.totp( base32Secret: enrollmentCode, // Enrollment code entered by user algorithm: .sha1 // Algorithm used by TOTP )
Was this helpful?
/生成されたコードを取得します。
codeGenerator.code()
Was this helpful?
/
ワンタイムコードを入力する
Auth0ログインプロンプトで、前のステップで生成したコードを入力します。
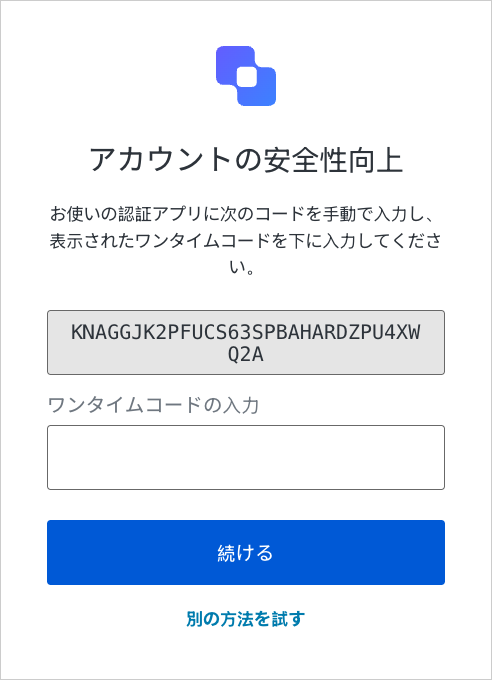
[Continue(続ける)]を選択すると、アプリケーションがユーザーの認証要素として追加されたことを示すメッセージが表示されます。
アプリでログインする
要素が登録されると、ユーザーはアプリを使用してログインできるようになります。まず、認証方法としてGuardianアプリを選択します。
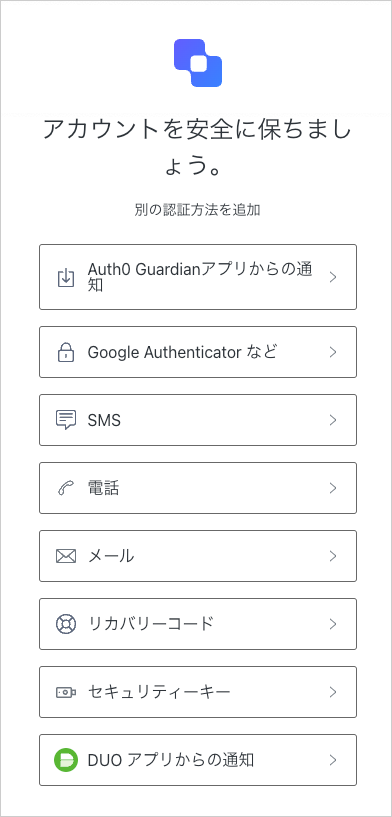
次に、ワンタイムコードをログインプロンプトに入力し、本人確認を行います。
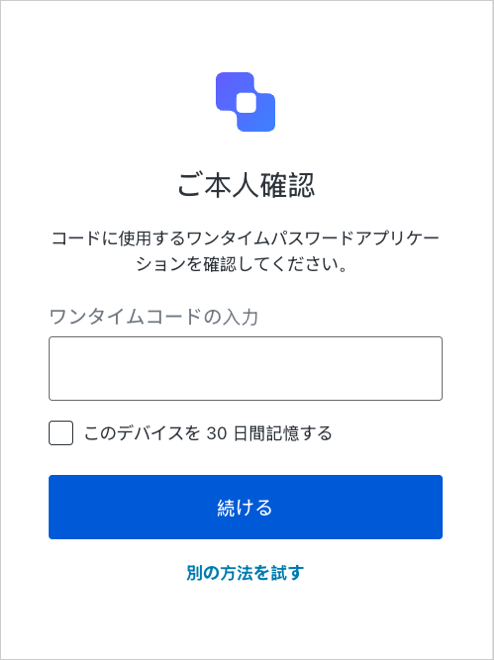