Revoke Refresh Tokens
You can revoke refresh tokens in case they become compromised. Auth0 handles token revocation as though the token has been potentially exposed to malicious adversaries.
You can also use refresh token rotation so that every time a client exchanges a refresh token to get a new access token, a new refresh token is also returned. Therefore, you no longer have a long-lived refresh token that, if compromised, could provide illegitimate access to resources. As refresh tokens are continually exchanged and invalidated, the threat is reduced.
You can revoke a refresh token in the following ways:
In the Dashboard
Post a request to the Authentication API
/oauth/revoke
endpointPost a request to the Management API
/api/v2/device-credentials
endpoint
Refresh tokens and grants
A grant provides an application with access to a resource on another entity without exposing user credentials. Tokens are issued in the context of a grant, and when a grant is revoked, so are all tokens issued in the context of that grant. When, on the other hand, a token is revoked, this does not necessarily mean that the grant is revoked.
You can choose the revocation behavior in the Dashboard tenant settings when a device is unlinked from a user in Auth0, using either the Dashboard or the Management API.
Go to Dashboard > Tenant Settings > Advanced and scroll to the Settings section.
Enable or disable the Refresh Token Revocation Deletes Grant toggle depending on how you want the revocation to work.
Enable the toggle to delete the underlying grant when you revoke the refresh token. Each revocation request invalidates not only the specific token but all other tokens based on the same authorization grant. This means that all refresh tokens that have been issued for the same user, application, and audience will be revoked.
Disable the toggle to retain the underlying grant when you revoke the refresh token. When a device is unlinked, only the associated refresh token is revoked, leaving the grant intact.
Use the Dashboard
You can use the Dashboard to revoke a user's authorized access to the application that issued the token. This renders the refresh token invalid, which is functionally identical to revoking the token itself.
Go to Dashboard > User Management > Users, and click the name of the user to view.
Select the Authorized Applications tab. This page lists all the applications to which the user has authorized access.
To revoke the user's access to an authorized application, and hence invalidate the refresh token, click Revoke.
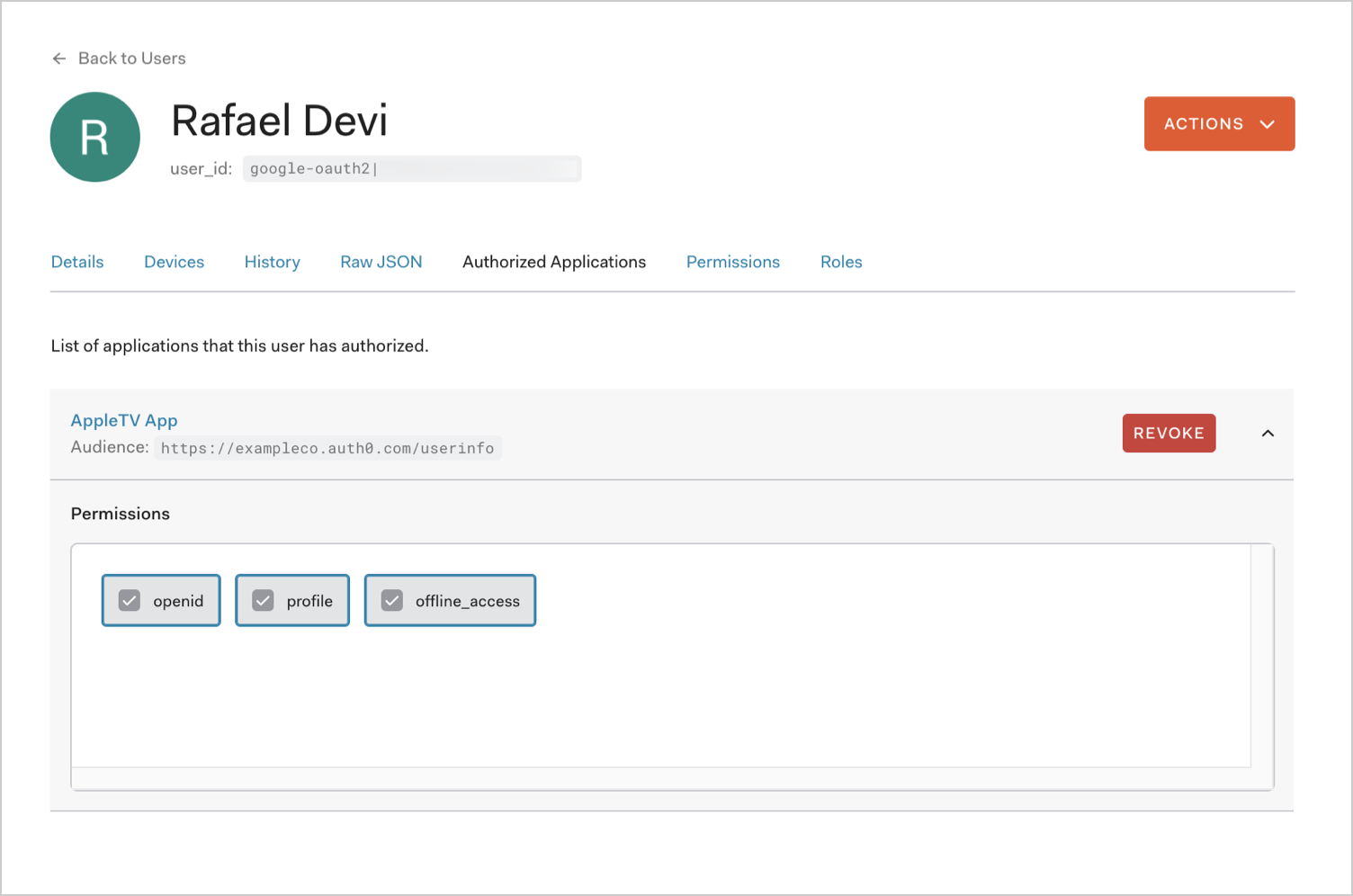
Use the Authentication API
To revoke a refresh token, send a POST
request to https://{yourDomain}/oauth/revoke
.
The /oauth/revoke
endpoint revokes the entire grant, not just a specific token. Use the /api/v2/device-credentials
endpoint to revoke refresh tokens. The API first validates the application credentials and then verifies whether the token was issued to the application making the revocation request. If this validation fails, the request is refused, and the application is informed of the error. Next, the API invalidates the token. The invalidation takes place immediately, and the token cannot be used again after the revocation. Each revocation request invalidates all the tokens that have been issued for the same authorization grant.
curl --request POST \
--url 'https://{yourDomain}/oauth/revoke' \
--header 'content-type: application/json' \
--data '{ "client_id": "{yourClientId}", "client_secret": "{yourClientSecret}", "token": "{yourRefreshToken}" }'
Was this helpful?
var client = new RestClient("https://{yourDomain}/oauth/revoke");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/json");
request.AddParameter("application/json", "{ \"client_id\": \"{yourClientId}\", \"client_secret\": \"{yourClientSecret}\", \"token\": \"{yourRefreshToken}\" }", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Was this helpful?
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://{yourDomain}/oauth/revoke"
payload := strings.NewReader("{ \"client_id\": \"{yourClientId}\", \"client_secret\": \"{yourClientSecret}\", \"token\": \"{yourRefreshToken}\" }")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
Was this helpful?
HttpResponse<String> response = Unirest.post("https://{yourDomain}/oauth/revoke")
.header("content-type", "application/json")
.body("{ \"client_id\": \"{yourClientId}\", \"client_secret\": \"{yourClientSecret}\", \"token\": \"{yourRefreshToken}\" }")
.asString();
Was this helpful?
var axios = require("axios").default;
var options = {
method: 'POST',
url: 'https://{yourDomain}/oauth/revoke',
headers: {'content-type': 'application/json'},
data: {
client_id: '{yourClientId}',
client_secret: '{yourClientSecret}',
token: '{yourRefreshToken}'
}
};
axios.request(options).then(function (response) {
console.log(response.data);
}).catch(function (error) {
console.error(error);
});
Was this helpful?
#import <Foundation/Foundation.h>
NSDictionary *headers = @{ @"content-type": @"application/json" };
NSDictionary *parameters = @{ @"client_id": @"{yourClientId}",
@"client_secret": @"{yourClientSecret}",
@"token": @"{yourRefreshToken}" };
NSData *postData = [NSJSONSerialization dataWithJSONObject:parameters options:0 error:nil];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://{yourDomain}/oauth/revoke"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setAllHTTPHeaderFields:headers];
[request setHTTPBody:postData];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"%@", httpResponse);
}
}];
[dataTask resume];
Was this helpful?
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://{yourDomain}/oauth/revoke",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => "{ \"client_id\": \"{yourClientId}\", \"client_secret\": \"{yourClientSecret}\", \"token\": \"{yourRefreshToken}\" }",
CURLOPT_HTTPHEADER => [
"content-type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Was this helpful?
import http.client
conn = http.client.HTTPSConnection("")
payload = "{ \"client_id\": \"{yourClientId}\", \"client_secret\": \"{yourClientSecret}\", \"token\": \"{yourRefreshToken}\" }"
headers = { 'content-type': "application/json" }
conn.request("POST", "/{yourDomain}/oauth/revoke", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Was this helpful?
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://{yourDomain}/oauth/revoke")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["content-type"] = 'application/json'
request.body = "{ \"client_id\": \"{yourClientId}\", \"client_secret\": \"{yourClientSecret}\", \"token\": \"{yourRefreshToken}\" }"
response = http.request(request)
puts response.read_body
Was this helpful?
import Foundation
let headers = ["content-type": "application/json"]
let parameters = [
"client_id": "{yourClientId}",
"client_secret": "{yourClientSecret}",
"token": "{yourRefreshToken}"
] as [String : Any]
let postData = JSONSerialization.data(withJSONObject: parameters, options: [])
let request = NSMutableURLRequest(url: NSURL(string: "https://{yourDomain}/oauth/revoke")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "POST"
request.allHTTPHeaderFields = headers
request.httpBody = postData as Data
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if (error != nil) {
print(error)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()
Was this helpful?
Where:
Attribute | Description |
---|---|
client_id Required |
Your application's Client ID. The application should match the one the Refresh Token was issued for. |
client_secret |
Your application's Client Secret. Required for confidential applications. |
token Required |
The Refresh Token you want to revoke. |
The application should match the one for which the refresh token was issued.
Revoke tokens without the client secret
For applications that cannot keep the client secret safe (such as native apps), the /oauth/revoke
endpoint supports access without the client secret. However, the application itself must have the property tokenEndpointAuthMethod
set to none
. You can change the tokenEndpointAuthMethod
value, either from the Dashboard > Applications > Applications, or using the Management API.
If the request is valid, the refresh token is revoked, and the response is HTTP 200
, with an empty response body. Otherwise, the response body contains the error code and description.
{
"error": "invalid_request|invalid_client",
"error_description": "Description of the error"
}
Was this helpful?
The possible responses are:
HTTP Status | Description |
---|---|
200 | The Refresh Token is revoked, does not exist, or was not issued to the application making the revocation request. The response body is empty. |
400 | The required parameters were not sent in the request ("error": "invalid_request" ). |
401 | The request is not authorized ("error": "invalid_client" ). Check that the application credentials (client_id and client_secret ) are present in the request and hold valid values. |
Use the Management API
To revoke a refresh token using the Auth0 Management API, you need the id
of the refresh token you wish to revoke. To obtain a list of existing refresh tokens, call the /api/v2/device-credentials
endpoint, specifying type=refresh_token
and user_id
with an access token containing read:device_credentials
scope. To narrow the results, you can also specify the client_id
associated with the token (if known).
GET https://{yourDomain}/api/v2/device-credentials?
type=refresh_token
&client_id=
&user_id=
{
"Authorization": "Bearer {your_access_token}"
}
Was this helpful?
Response body:
[
{
"id": "dcr_dFJiaAxbEroQ5xxx",
"device_name": "my-device" // the value of 'device' provided in the /authorize call when creating the token
}
]
Was this helpful?
To revoke a refresh token, call the /api/v2/device-credentials
endpoint with an access token containing delete:device_credentials
scope and the value of ID obtained above:
DELETE https://{yourDomain}/api/v2/device-credentials/{id}
{
"Authorization": "Bearer {your_access_token}"
}
Was this helpful?
The response will be HTTP 204: The credential no longer exists.
Considerations and limitations
With the Device Authorization Flow, the only way to force a device to reauthorize is to revoke the refresh token assigned to the device. See Unlink Devices from Users for details. The device will not be forced to reauthorize until the current access token expires and the application tries to use the revoked refresh token.
When using Refresh Token Rotation, if a previously invalidated token is used, the entire set of refresh tokens issued since that invalidated token was issued will immediately be revoked, requiring the end-user to re-authenticate.
Use the Authentication API
/oauth/revoke
endpoint to revoke a refresh token. This endpoint does not delete the underlying grant. You can change this behavior to also delete the underlying grant in the Dashboard: Dashboard > Tenant Settings > Advanced. Scroll to Settings and enable the Refresh Token Revocation Deletes Grant toggle.Use the Management API
/api/v2/device-credentials
endpoint to revoke refresh tokens configured for rotation.