Connect Your App to SAML Identity Providers
Auth0 lets you create SAML Identity Provider (IdP) connections.
Prerequisites
Before beginning:
Register your Application with Auth0.
Select an appropriate Application Type.
Add an Allowed Callback URL of
{https://yourApp/callback}
.Make sure your Application's Grant Types include the appropriate flows.
Decide on the name of this enterprise connection
The Post-back URL (also called Assertion Consumer Service URL) becomes:
https://{yourDomain}/login/callback?connection={yourConnectionName}
The Entity ID becomes:
urn:auth0:{yourTenant}:{yourConnectionName}
Steps
To connect your application to a SAML Identity Provider, you must:
Enter the Post-back URL and Entity ID at the IdP (to learn how, read about SAML Identity Provider Configuration Settings).
Get the signing certificate from the IdP and convert it to Base64.
Enable the enterprise connection for your Auth0 Application.
Set up mappings (unnecessary for most cases).
Get the signing certificate from the IdP
With SAML Login, Auth0 acts as the service provider, so you will need to retrieve an X.509 signing certificate from the SAML IdP (in PEM or CER format); later, you will upload this to Auth0. The methods for retrieving this certificate vary, so please see your IdP's documentation if you need additional assistance.
Convert signing certificate to Base64
You can use the Management API or the Auth0 Dashboard to upload the X.509 signing certificate. If you use the Management API, you must convert the file to Base64. To do this, either use a simple online tool or run the following command in Bash: cat signing-cert.crt | base64
.
Create an enterprise connection in Auth0
Next, you will need to create and configure a SAML Enterprise Connection in Auth0 and upload your X.509 signing certificate. This task can be performed using either Auth0's Dashboard or Management API.
Create an enterprise connection using the Dashboard
Navigate to Auth0 Dashboard > Authentication > Enterprise, locate SAML, and select its
+
.Enter details for your connection, and select Create:
Field Description Connection name Logical identifier for your connection; it must be unique for your tenant and the same name used when setting the Post-back URL and Entity ID at the IdP. Once set, this name can't be changed. Sign In URL SAML single login URL. X.509 Signing Certificate Signing certificate (encoded in PEM or CER) you retrieved from the IdP earlier in this process. Enable Sign Out When enabled, a specific Sign Out URL can be set. Otherwise, the Sign In URL is used by default. Sign Out URL (optional) SAML single logout URL. User ID Attribute (optional) Attribute in the SAML token that will be mapped to the user_id
property in Auth0.Debug Mode When enabled, more verbose logging will be performed during the authentication process. Sign Request When enabled, the SAML authentication request will be signed. (Be sure to download and provide the accompanying certificate so the SAML IdP can validate the assertions' signature.) Sign Request Algorithm Algorithm Auth0 will use to sign the SAML assertions. Sign Request Digest Algorithm Algorithm Auth0 will use for the sign request digest. Protocol Binding HTTP binding supported by the IdP. Request Template (optional) Template that formats the SAML request. In the Provisioning view, configure how user profiles get created and updated in Auth0.
Field Description Sync user profile attributes at each login When enabled, Auth0 automatically syncs user profile data with each user login, thereby ensuring that changes made in the connection source are automatically updated in Auth0. Sync user profiles using SCIM When enabled, Auth0 allows user profile data to be synced using SCIM. For more information, see Configure Inbound SCIM. In the Login Experience view, configure how users log in with this connection.
Field Description Home Realm Discovery Compares a user's email domain with the provided identity provider domains. For more information, read Configure Identifier First Authentication Display connection button This option displays the following choices to customize your application's connection button. Button display name (Optional) Text used to customize the login button for Universal Login. When set the button reads: "Continue with {Button display name}". Button logo URL (Optional) URL of image used to customize the login button for Universal Login. When set, the Universal Login login button displays the image as a 20px by 20px square. If you have appropriate administrative permissions to complete the integration, click Continue to learn about the custom parameters needed to configure your IdP. Otherwise, provide the given URL to your administrator so that they can adjust the required settings.
Create an enterprise connection using the Management API
You can also use the Management API to create your SAML Connection. When doing so, you may choose to specify each SAML configuration field manually or else specify a SAML metadata document that contains the configuration values.
Create a connection using specified values
Make a POST
call to the Create a Connection endpoint. Be sure to replace MGMT_API_ACCESS_TOKEN
, CONNECTION_NAME
, SIGN_IN_ENDPOINT_URL
, SIGN_OUT_ENDPOINT_URL
, and BASE64_SIGNING_CERT
placeholder values with your Management API Access Token, connection name, sign in URL, sign out URL, and Base64-encoded signing certificate (in PEM or CER format), respectively.
curl --request POST \
--url 'https://{yourDomain}/api/v2/connections' \
--header 'authorization: Bearer MGMT_API_ACCESS_TOKEN' \
--header 'cache-control: no-cache' \
--header 'content-type: application/json' \
--data '{ "strategy": "samlp", "name": "CONNECTION_NAME", "options": { "signInEndpoint": "SIGN_IN_ENDPOINT_URL", "signOutEndpoint": "SIGN_OUT_ENDPOINT_URL", "signatureAlgorithm": "rsa-sha256", "digestAlgorithm": "sha256", "fieldsMap": {}, "signingCert": "BASE64_SIGNING_CERT" } }'
Was this helpful?
var client = new RestClient("https://{yourDomain}/api/v2/connections");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/json");
request.AddHeader("authorization", "Bearer MGMT_API_ACCESS_TOKEN");
request.AddHeader("cache-control", "no-cache");
request.AddParameter("application/json", "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"signInEndpoint\": \"SIGN_IN_ENDPOINT_URL\", \"signOutEndpoint\": \"SIGN_OUT_ENDPOINT_URL\", \"signatureAlgorithm\": \"rsa-sha256\", \"digestAlgorithm\": \"sha256\", \"fieldsMap\": {}, \"signingCert\": \"BASE64_SIGNING_CERT\" } }", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Was this helpful?
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://{yourDomain}/api/v2/connections"
payload := strings.NewReader("{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"signInEndpoint\": \"SIGN_IN_ENDPOINT_URL\", \"signOutEndpoint\": \"SIGN_OUT_ENDPOINT_URL\", \"signatureAlgorithm\": \"rsa-sha256\", \"digestAlgorithm\": \"sha256\", \"fieldsMap\": {}, \"signingCert\": \"BASE64_SIGNING_CERT\" } }")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("content-type", "application/json")
req.Header.Add("authorization", "Bearer MGMT_API_ACCESS_TOKEN")
req.Header.Add("cache-control", "no-cache")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
Was this helpful?
HttpResponse<String> response = Unirest.post("https://{yourDomain}/api/v2/connections")
.header("content-type", "application/json")
.header("authorization", "Bearer MGMT_API_ACCESS_TOKEN")
.header("cache-control", "no-cache")
.body("{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"signInEndpoint\": \"SIGN_IN_ENDPOINT_URL\", \"signOutEndpoint\": \"SIGN_OUT_ENDPOINT_URL\", \"signatureAlgorithm\": \"rsa-sha256\", \"digestAlgorithm\": \"sha256\", \"fieldsMap\": {}, \"signingCert\": \"BASE64_SIGNING_CERT\" } }")
.asString();
Was this helpful?
var axios = require("axios").default;
var options = {
method: 'POST',
url: 'https://{yourDomain}/api/v2/connections',
headers: {
'content-type': 'application/json',
authorization: 'Bearer MGMT_API_ACCESS_TOKEN',
'cache-control': 'no-cache'
},
data: {
strategy: 'samlp',
name: 'CONNECTION_NAME',
options: {
signInEndpoint: 'SIGN_IN_ENDPOINT_URL',
signOutEndpoint: 'SIGN_OUT_ENDPOINT_URL',
signatureAlgorithm: 'rsa-sha256',
digestAlgorithm: 'sha256',
fieldsMap: {},
signingCert: 'BASE64_SIGNING_CERT'
}
}
};
axios.request(options).then(function (response) {
console.log(response.data);
}).catch(function (error) {
console.error(error);
});
Was this helpful?
#import <Foundation/Foundation.h>
NSDictionary *headers = @{ @"content-type": @"application/json",
@"authorization": @"Bearer MGMT_API_ACCESS_TOKEN",
@"cache-control": @"no-cache" };
NSDictionary *parameters = @{ @"strategy": @"samlp",
@"name": @"CONNECTION_NAME",
@"options": @{ @"signInEndpoint": @"SIGN_IN_ENDPOINT_URL", @"signOutEndpoint": @"SIGN_OUT_ENDPOINT_URL", @"signatureAlgorithm": @"rsa-sha256", @"digestAlgorithm": @"sha256", @"fieldsMap": @{ }, @"signingCert": @"BASE64_SIGNING_CERT" } };
NSData *postData = [NSJSONSerialization dataWithJSONObject:parameters options:0 error:nil];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://{yourDomain}/api/v2/connections"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setAllHTTPHeaderFields:headers];
[request setHTTPBody:postData];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"%@", httpResponse);
}
}];
[dataTask resume];
Was this helpful?
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://{yourDomain}/api/v2/connections",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"signInEndpoint\": \"SIGN_IN_ENDPOINT_URL\", \"signOutEndpoint\": \"SIGN_OUT_ENDPOINT_URL\", \"signatureAlgorithm\": \"rsa-sha256\", \"digestAlgorithm\": \"sha256\", \"fieldsMap\": {}, \"signingCert\": \"BASE64_SIGNING_CERT\" } }",
CURLOPT_HTTPHEADER => [
"authorization: Bearer MGMT_API_ACCESS_TOKEN",
"cache-control: no-cache",
"content-type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Was this helpful?
import http.client
conn = http.client.HTTPSConnection("")
payload = "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"signInEndpoint\": \"SIGN_IN_ENDPOINT_URL\", \"signOutEndpoint\": \"SIGN_OUT_ENDPOINT_URL\", \"signatureAlgorithm\": \"rsa-sha256\", \"digestAlgorithm\": \"sha256\", \"fieldsMap\": {}, \"signingCert\": \"BASE64_SIGNING_CERT\" } }"
headers = {
'content-type': "application/json",
'authorization': "Bearer MGMT_API_ACCESS_TOKEN",
'cache-control': "no-cache"
}
conn.request("POST", "/{yourDomain}/api/v2/connections", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Was this helpful?
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://{yourDomain}/api/v2/connections")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["content-type"] = 'application/json'
request["authorization"] = 'Bearer MGMT_API_ACCESS_TOKEN'
request["cache-control"] = 'no-cache'
request.body = "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"signInEndpoint\": \"SIGN_IN_ENDPOINT_URL\", \"signOutEndpoint\": \"SIGN_OUT_ENDPOINT_URL\", \"signatureAlgorithm\": \"rsa-sha256\", \"digestAlgorithm\": \"sha256\", \"fieldsMap\": {}, \"signingCert\": \"BASE64_SIGNING_CERT\" } }"
response = http.request(request)
puts response.read_body
Was this helpful?
import Foundation
let headers = [
"content-type": "application/json",
"authorization": "Bearer MGMT_API_ACCESS_TOKEN",
"cache-control": "no-cache"
]
let parameters = [
"strategy": "samlp",
"name": "CONNECTION_NAME",
"options": [
"signInEndpoint": "SIGN_IN_ENDPOINT_URL",
"signOutEndpoint": "SIGN_OUT_ENDPOINT_URL",
"signatureAlgorithm": "rsa-sha256",
"digestAlgorithm": "sha256",
"fieldsMap": [],
"signingCert": "BASE64_SIGNING_CERT"
]
] as [String : Any]
let postData = JSONSerialization.data(withJSONObject: parameters, options: [])
let request = NSMutableURLRequest(url: NSURL(string: "https://{yourDomain}/api/v2/connections")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "POST"
request.allHTTPHeaderFields = headers
request.httpBody = postData as Data
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if (error != nil) {
print(error)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()
Was this helpful?
Value | Description |
---|---|
MGMT_API_ACCESS_TOKEN |
Access Token for the Management API with the scope create:connections . |
CONNECTION_NAME |
Τhe name of the connection to be created. |
SIGN_IN_ENDPONT_URL |
SAML single login URL for the connection to be created. |
SIGN_OUT_ENDPOINT_URL |
SAML single logout URL for the connection to be created. |
BASE64_SIGNING_CERT |
X.509 signing certificate (encoded in PEM or CER) you retrieved from the IdP. |
Or, in JSON:
{
"strategy": "samlp",
"name": "CONNECTION_NAME",
"options": {
"signInEndpoint": "SIGN_IN_ENDPOINT_URL",
"signOutEndpoint": "SIGN_OUT_ENDPOINT_URL",
"signatureAlgorithm": "rsa-sha256",
"digestAlgorithm": "sha256",
"fieldsMap": {
...
},
"signingCert": "BASE64_SIGNING_CERT"
}
}
Was this helpful?
Create a connection using SAML metadata
Rather than specifying each SAML configuration field, you can specify a SAML metadata document that contains the configuration values. When specifying a SAML metadata document, you may provide either the XML content of the document (metadataXml
) or the URL of the document (metadataUrl
). When providing the URL, content is downloaded only once; the connection will not automatically reconfigure if the content of the URL changes in the future.
Provide metadata document content
Use the metadataXml
option to provide content of the document:
curl --request POST \
--url 'https://{yourDomain}/api/v2/connections' \
--header 'authorization: Bearer MGMT_API_ACCESS_TOKEN' \
--header 'cache-control: no-cache' \
--header 'content-type: application/json' \
--data '{ "strategy": "samlp", "name": "CONNECTION_NAME", "options": { "metadataXml": "<EntityDescriptor entityID='\''urn:saml-idp'\'' xmlns='\''urn:oasis:names:tc:SAML:2.0:metadata'\''>...</EntityDescriptor>" } }'
Was this helpful?
var client = new RestClient("https://{yourDomain}/api/v2/connections");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/json");
request.AddHeader("authorization", "Bearer MGMT_API_ACCESS_TOKEN");
request.AddHeader("cache-control", "no-cache");
request.AddParameter("application/json", "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataXml\": \"<EntityDescriptor entityID='urn:saml-idp' xmlns='urn:oasis:names:tc:SAML:2.0:metadata'>...</EntityDescriptor>\" } }", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Was this helpful?
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://{yourDomain}/api/v2/connections"
payload := strings.NewReader("{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataXml\": \"<EntityDescriptor entityID='urn:saml-idp' xmlns='urn:oasis:names:tc:SAML:2.0:metadata'>...</EntityDescriptor>\" } }")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("content-type", "application/json")
req.Header.Add("authorization", "Bearer MGMT_API_ACCESS_TOKEN")
req.Header.Add("cache-control", "no-cache")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
Was this helpful?
HttpResponse<String> response = Unirest.post("https://{yourDomain}/api/v2/connections")
.header("content-type", "application/json")
.header("authorization", "Bearer MGMT_API_ACCESS_TOKEN")
.header("cache-control", "no-cache")
.body("{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataXml\": \"<EntityDescriptor entityID='urn:saml-idp' xmlns='urn:oasis:names:tc:SAML:2.0:metadata'>...</EntityDescriptor>\" } }")
.asString();
Was this helpful?
var axios = require("axios").default;
var options = {
method: 'POST',
url: 'https://{yourDomain}/api/v2/connections',
headers: {
'content-type': 'application/json',
authorization: 'Bearer MGMT_API_ACCESS_TOKEN',
'cache-control': 'no-cache'
},
data: {
strategy: 'samlp',
name: 'CONNECTION_NAME',
options: {
metadataXml: '<EntityDescriptor entityID=\'urn:saml-idp\' xmlns=\'urn:oasis:names:tc:SAML:2.0:metadata\'>...</EntityDescriptor>'
}
}
};
axios.request(options).then(function (response) {
console.log(response.data);
}).catch(function (error) {
console.error(error);
});
Was this helpful?
#import <Foundation/Foundation.h>
NSDictionary *headers = @{ @"content-type": @"application/json",
@"authorization": @"Bearer MGMT_API_ACCESS_TOKEN",
@"cache-control": @"no-cache" };
NSDictionary *parameters = @{ @"strategy": @"samlp",
@"name": @"CONNECTION_NAME",
@"options": @{ @"metadataXml": @"<EntityDescriptor entityID='urn:saml-idp' xmlns='urn:oasis:names:tc:SAML:2.0:metadata'>...</EntityDescriptor>" } };
NSData *postData = [NSJSONSerialization dataWithJSONObject:parameters options:0 error:nil];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://{yourDomain}/api/v2/connections"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setAllHTTPHeaderFields:headers];
[request setHTTPBody:postData];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"%@", httpResponse);
}
}];
[dataTask resume];
Was this helpful?
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://{yourDomain}/api/v2/connections",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataXml\": \"<EntityDescriptor entityID='urn:saml-idp' xmlns='urn:oasis:names:tc:SAML:2.0:metadata'>...</EntityDescriptor>\" } }",
CURLOPT_HTTPHEADER => [
"authorization: Bearer MGMT_API_ACCESS_TOKEN",
"cache-control: no-cache",
"content-type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Was this helpful?
import http.client
conn = http.client.HTTPSConnection("")
payload = "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataXml\": \"<EntityDescriptor entityID='urn:saml-idp' xmlns='urn:oasis:names:tc:SAML:2.0:metadata'>...</EntityDescriptor>\" } }"
headers = {
'content-type': "application/json",
'authorization': "Bearer MGMT_API_ACCESS_TOKEN",
'cache-control': "no-cache"
}
conn.request("POST", "/{yourDomain}/api/v2/connections", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Was this helpful?
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://{yourDomain}/api/v2/connections")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["content-type"] = 'application/json'
request["authorization"] = 'Bearer MGMT_API_ACCESS_TOKEN'
request["cache-control"] = 'no-cache'
request.body = "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataXml\": \"<EntityDescriptor entityID='urn:saml-idp' xmlns='urn:oasis:names:tc:SAML:2.0:metadata'>...</EntityDescriptor>\" } }"
response = http.request(request)
puts response.read_body
Was this helpful?
import Foundation
let headers = [
"content-type": "application/json",
"authorization": "Bearer MGMT_API_ACCESS_TOKEN",
"cache-control": "no-cache"
]
let parameters = [
"strategy": "samlp",
"name": "CONNECTION_NAME",
"options": ["metadataXml": "<EntityDescriptor entityID='urn:saml-idp' xmlns='urn:oasis:names:tc:SAML:2.0:metadata'>...</EntityDescriptor>"]
] as [String : Any]
let postData = JSONSerialization.data(withJSONObject: parameters, options: [])
let request = NSMutableURLRequest(url: NSURL(string: "https://{yourDomain}/api/v2/connections")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "POST"
request.allHTTPHeaderFields = headers
request.httpBody = postData as Data
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if (error != nil) {
print(error)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()
Was this helpful?
Provide a metadata document URL
Use the metadataUrl
option to provide the URL of the document:
curl --request POST \
--url 'https://{yourDomain}/api/v2/connections' \
--header 'authorization: Bearer MGMT_API_ACCESS_TOKEN' \
--header 'cache-control: no-cache' \
--header 'content-type: application/json' \
--data '{ "strategy": "samlp", "name": "CONNECTION_NAME", "options": { "metadataUrl": "https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX" } }'
Was this helpful?
var client = new RestClient("https://{yourDomain}/api/v2/connections");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/json");
request.AddHeader("authorization", "Bearer MGMT_API_ACCESS_TOKEN");
request.AddHeader("cache-control", "no-cache");
request.AddParameter("application/json", "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataUrl\": \"https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX\" } }", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Was this helpful?
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://{yourDomain}/api/v2/connections"
payload := strings.NewReader("{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataUrl\": \"https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX\" } }")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("content-type", "application/json")
req.Header.Add("authorization", "Bearer MGMT_API_ACCESS_TOKEN")
req.Header.Add("cache-control", "no-cache")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
Was this helpful?
HttpResponse<String> response = Unirest.post("https://{yourDomain}/api/v2/connections")
.header("content-type", "application/json")
.header("authorization", "Bearer MGMT_API_ACCESS_TOKEN")
.header("cache-control", "no-cache")
.body("{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataUrl\": \"https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX\" } }")
.asString();
Was this helpful?
var axios = require("axios").default;
var options = {
method: 'POST',
url: 'https://{yourDomain}/api/v2/connections',
headers: {
'content-type': 'application/json',
authorization: 'Bearer MGMT_API_ACCESS_TOKEN',
'cache-control': 'no-cache'
},
data: {
strategy: 'samlp',
name: 'CONNECTION_NAME',
options: {
metadataUrl: 'https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX'
}
}
};
axios.request(options).then(function (response) {
console.log(response.data);
}).catch(function (error) {
console.error(error);
});
Was this helpful?
#import <Foundation/Foundation.h>
NSDictionary *headers = @{ @"content-type": @"application/json",
@"authorization": @"Bearer MGMT_API_ACCESS_TOKEN",
@"cache-control": @"no-cache" };
NSDictionary *parameters = @{ @"strategy": @"samlp",
@"name": @"CONNECTION_NAME",
@"options": @{ @"metadataUrl": @"https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX" } };
NSData *postData = [NSJSONSerialization dataWithJSONObject:parameters options:0 error:nil];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://{yourDomain}/api/v2/connections"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setAllHTTPHeaderFields:headers];
[request setHTTPBody:postData];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"%@", httpResponse);
}
}];
[dataTask resume];
Was this helpful?
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://{yourDomain}/api/v2/connections",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataUrl\": \"https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX\" } }",
CURLOPT_HTTPHEADER => [
"authorization: Bearer MGMT_API_ACCESS_TOKEN",
"cache-control: no-cache",
"content-type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Was this helpful?
import http.client
conn = http.client.HTTPSConnection("")
payload = "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataUrl\": \"https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX\" } }"
headers = {
'content-type': "application/json",
'authorization': "Bearer MGMT_API_ACCESS_TOKEN",
'cache-control': "no-cache"
}
conn.request("POST", "/{yourDomain}/api/v2/connections", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Was this helpful?
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://{yourDomain}/api/v2/connections")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["content-type"] = 'application/json'
request["authorization"] = 'Bearer MGMT_API_ACCESS_TOKEN'
request["cache-control"] = 'no-cache'
request.body = "{ \"strategy\": \"samlp\", \"name\": \"CONNECTION_NAME\", \"options\": { \"metadataUrl\": \"https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX\" } }"
response = http.request(request)
puts response.read_body
Was this helpful?
import Foundation
let headers = [
"content-type": "application/json",
"authorization": "Bearer MGMT_API_ACCESS_TOKEN",
"cache-control": "no-cache"
]
let parameters = [
"strategy": "samlp",
"name": "CONNECTION_NAME",
"options": ["metadataUrl": "https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX"]
] as [String : Any]
let postData = JSONSerialization.data(withJSONObject: parameters, options: [])
let request = NSMutableURLRequest(url: NSURL(string: "https://{yourDomain}/api/v2/connections")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "POST"
request.allHTTPHeaderFields = headers
request.httpBody = postData as Data
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if (error != nil) {
print(error)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()
Was this helpful?
When providing the URL, content is downloaded only once; the connection will not automatically reconfigure if the content of the URL changes in the future.
Refresh existing connection information with metadata URL
If you have a B2B implementation and federate to Auth0 with your own SAML identity provider, you may need to refresh connection information stored in Auth0, such as signing certificate changes, endpoint URL changes, or new assertion fields. Auth0 does this automatically for ADFS connections, but not for SAML connections.
You can create a batch process (cron job) to do a periodic refresh. The process can run every few weeks and perform a PATCH call to /api/v2/connections/CONNECTION_ID
endpoint, passing a body containing {options: {metadataUrl: '$URL'}}
where $URL
is the same metadata URL with which you created the connection. You use the metadata URL to create a new temporary connection, then compare the properties of the old and new connections. If anything is different, update the new connection and then delete the temporary connection.
Create SAML connection with
options.metadataUrl
. The connection object will be populated with information from the metadata.Update metadata content in the URL.
Send a PATCH to the
/api/v2/connections/CONNECTION_ID
endpoint with{options: {metadataUrl: '$URL'}}
. Now the connection object is updated with the new metadata content.
Specify a custom Entity ID
To specify a custom Entity ID, use the Management API to override the default urn:auth0:YOUR_TENANT:YOUR_CONNECTION_NAME
. Set the connection.options.entityID
property when the connection is first created or by updating an existing connection.
The JSON example below can be used to create a new SAML connection using the SAML IdP’s metadata URL while also specifying a custom Entity ID. The Entity ID is still unique since it is created using the name of the connection.
{
"strategy": "samlp",
"name": "{yourConnectionName}",
"options": {
"metadataUrl": "https://saml-idp/samlp/metadata/uarlU13n63e0feZNJxOCNZ1To3a9H7jX",
"entityId": "urn:your-custom-sp-name:{yourConnectionName}"
}
}
Was this helpful?
Enable the enterprise connection for your Auth0 application
To use your new SAML enterprise connection, you must first enable the connection for your Auth0 Applications.
Set up mappings
Select the Mappings view, enter mappings between the {}
, and select Save.
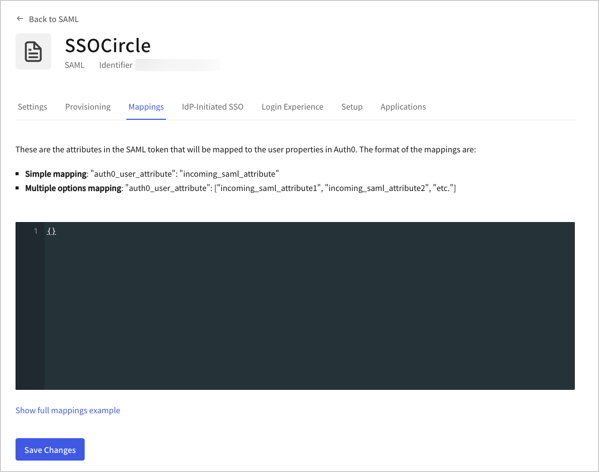
Mappings for non-standard PingFederate Servers:
{
"user_id": "http://schemas.xmlsoap.org/ws/2005/05/identity/claims/nameidentifier",
"email": "http://schemas.xmlsoap.org/ws/2005/05/identity/claims/nameidentifier"
}
Was this helpful?
Mappings for SSO Circle
{
"email": "EmailAddress",
"given_name": "FirstName",
"family_name": "LastName"
}
Was this helpful?
Map either of two claims to one user attribute
{
"given_name": [
"http://schemas.xmlsoap.org/ws/2005/05/identity/claims/givenname",
"http://schemas.xmlsoap.org/ws/2005/05/identity/claims/name"
]
}
Was this helpful?
How to map name identifier to a user attribute
{
"user_id": [
"http://schemas.xmlsoap.org/ws/2005/05/identity/claims/nameidentifier",
"http://schemas.xmlsoap.org/ws/2005/05/identity/claims/upn",
"http://schemas.xmlsoap.org/ws/2005/05/identity/claims/name"
]
}
Was this helpful?
Test the connection
Now you're ready to test your connection.
Configure Global Token Revocation
This connection type supports a Global Token Revocation endpoint, which allows a compliant identity provider to revoke Auth0 user sessions, revoke refresh tokens, and trigger back-channel logout for applications using a secure back-channel.
This feature can be used with Universal Logout in Okta Workforce Identity.
For more information and configuration instructions, see Universal Logout.