Advanced Customizations for Universal Login
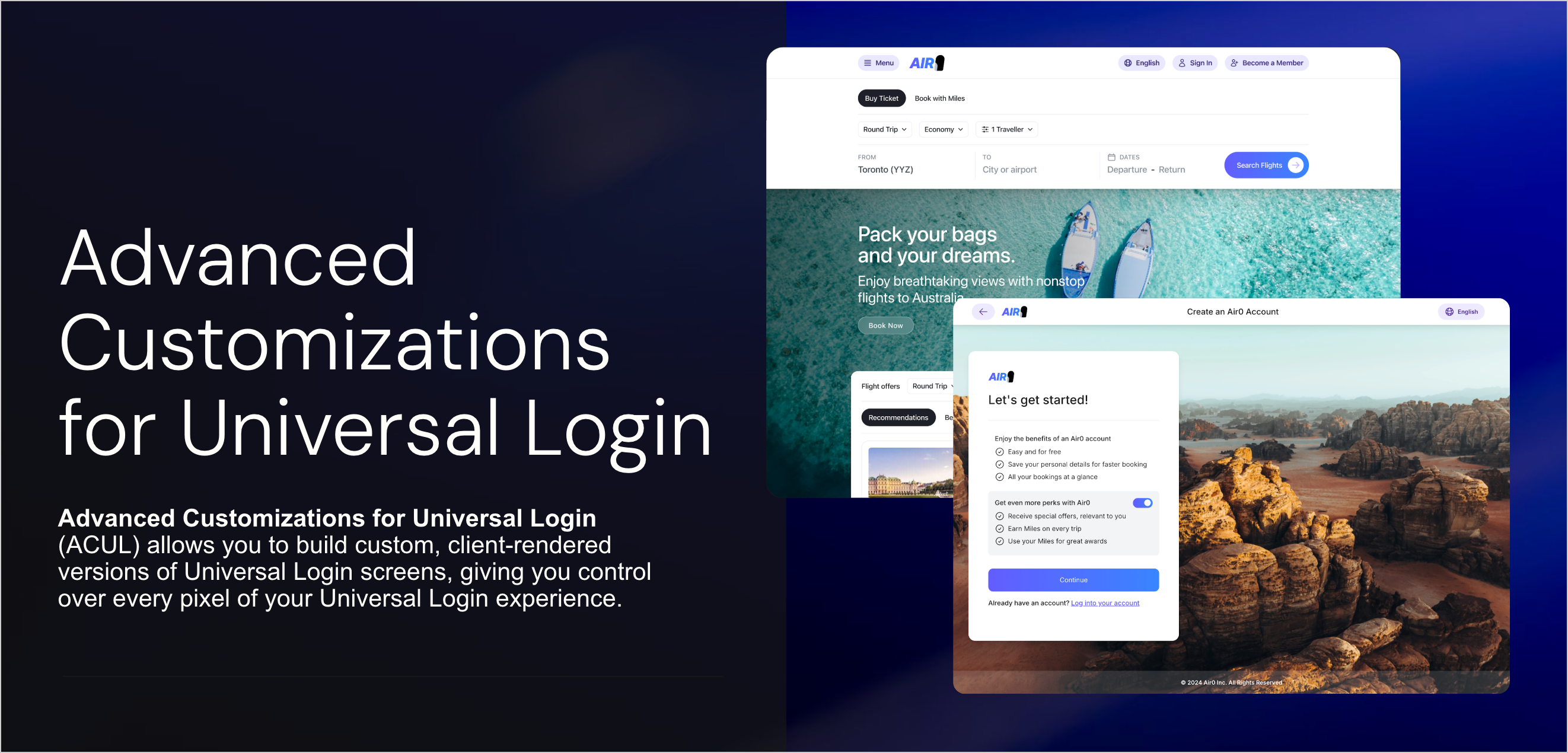
Advanced Customizations for Universal Login (ACUL) is an Early Access capability for Universal Login that allows you to build custom, client-rendered versions of Universal Login screens, giving you control over every pixel of your Universal Login experience. Advanced Customizations for Universal Login can help your organization:
Create pixel-perfect branding: Design user interfaces that look and feel a part of your brand.
Support multiple brands or customers in one tenant: Leverage application, user, and organization information to deliver multi-brand authentication experiences.
Gather advanced analytics & metrics: Integrate A/B testing and analytics platforms to optimize and innovate on onboarding journeys.
Comply with regional requirements: Follow region-specific requirements around localization, privacy, and accessibility.
Leverage existing technology investments: Utilize the technologies that already power your applications, design-systems, CSS, and Javascript frameworks.
Build custom authentication experiences secured by Auth0: Connect the security and extensibility of the Auth0 features like passkeys, threat intelligence, and Security Center with your organization's aesthetics and user experience.
Balance the effort & impact of front-end development: Iteratively or selectively adopt ACUL based on your company’s specific business needs and use cases.
Advanced Customizations for Universal Login is built on top of the core capabilities of Auth0. It uses a client/server model to give you full control over the client-side interface while leveraging the security, extensibility, and flexibility of Universal Login’s hosted authentication on the server side. Capabilities available in Universal Login work out of the box with Advanced Customizations for Universal Login.
To begin using Advanced Customizations for Universal Login, review Getting Started with Advanced Customization.
What is supported in Early Access?
During the Early Access phase, Auth0 will release and support custom interfaces for every screen and capability included by default in Universal Login.
Early Access is supported for use in Production, and future EA releases will be non-breaking, meaning they will be backward compatible with existing supported screens and features. Below is a list of the currently supported screens and capabilities; this information can also be found in the Auth0 Changelog.
This EA release focuses on the configuration API, dev tooling, basic SDK support, and building custom UIs for the first factor screens and capabilities related to the Identifier First authentication profile, including:
Management API endpoints for configuring Advanced Customizations for Universal Login on a per screen basis
Support for the new Management API endpoints in Terraform Provider, Deploy CLI, and Auth0 CLI
A Typescript SDK for building interfaces and interacting with the server
Support building custom UIs for the ID First first-factor screens
Supported authentication methods
Password
Passwordless Email/SMS OTP
Passkeys
Enterprise/Social Connections
Supported client-side capabilities
Flexible Identifiers
Bot Detection (Captcha)
Capturing additional data during login & signup
Supported screens:
This release enables ACUL, by default, for all tenants on a paid plan and focuses on building custom UIs for the single step signup/login flows and the basic reset password flow, including:
signup
login
reset-password
reset-password-email
reset-password-request
reset-password-error
reset-password-success
It also includes updates to the Javascript SDK and our CDT tooling to support these new screens.
This release adds support for user-built custom versions of Universal Login’s multi-factor authentication enrollment screens and three of our most common MFA factors using the new ACUL SDK, including:
mfa-detect-browser-capabilities
mfa-begin-enroll-options
mfa-enroll-result
mfa-login-options
mfa-email-list
mfa-email-challenge
mfa-country-codes
mfa-sms-enrollment
mfa-sms-list
mfa-sms-challenge
mfa-push-welcome
mfa-push-enrollment-qr
mfa-push-list
mfa-push-challenge-push
This release adds support for custom versions of Universal Login’s Organizations screens, multi-factor authentication (MFA) time-based one-time password screens, and each MFA factor's matching Reset Password Challenge factors using the new ACUL SDK, including:
Accept Invitation
Organization Selection
Organization Picker
MFA OTP Enrollment QR
MFA OTP Enrollment Code
MFA OTP Challenge
Reset Password MFA OTP Challenge
Reset Password MFA Email Challenge
Reset Password MFA Push Challenge Push
Reset Password MFA SMS Challenge
DX Updates The latest versions of the ACUL SDK and our CDT tooling include support for these new screens.
Typescript SDK (includes a breaking change; the changelog in GitHub has migration steps)
Date: April 28, 2025
This release adds support for building custom versions of Universal Login’s Device Authorization screens, MFA voice, phone, and recovery code screens, and matching Reset Password Challenge factors, all of which use the new ACUL SDK. The following screens are new:
Device Code Activation
Device Code Activation Allowed
Device Code Activation Denied
Device Code Confirmation
MFA Phone Challenge
MFA Phone Enrollment
MFA Voice Challenge
MFA Voice Enrollment
Reset Password MFA Phone Challenge
Reset Password MFA Voice Challenge
MFA Recovery Code Challenge
MFA Recovery Code Enrollment
Reset Password MFA Recovery Code Challenge
Redeem Ticket
DX Updates
The latest versions of the ACUL SDK and our CDT tooling all include support for these new screens.
Date: May 27, 2025
This release adds support for building custom versions of Universal Login’s WebAuthn + Biometrics authentication and the matching MFA and Reset Password Challenge screens, as well as Logout and a few others, all of which use the new ACUL SDK. The following screens are new:
MFA WebAuthn Change Key Nickname
MFA WebAuthn Enrollment Success
MFA WebAuthn Error
MFA WebAuthn Platform Challenge
MFA WebAuthn Platform Enrollment
MFA WebAuthn Roaming Challenge
MFA WebAuthn Roaming Enrollment
Reset Password MFA WebAuthn Platform Challenge
Reset Password MFA WebAuthn Roaming Challenge
Logout
Logout Aborted
Logout Complete
MFA Recovery Code Challenge New Code
Email Verification Result
Login Email Verification
DX Updates
The latest versions of the ACUL SDK and our CDT tooling all include support for these new screens.