Interstitial Captcha screen class
The Interstitial Captcha screen class is part of the Identifier First Authentication flow and is presented based on the tenant settings. For more details, review Bot Detection.
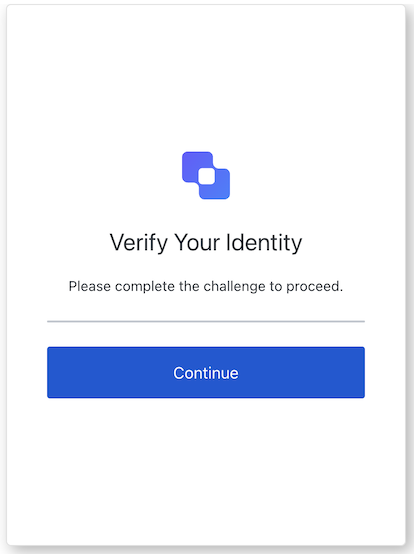
Import and instantiate the Interstitial Captcha screen class:
import InterstitialCaptcha from "@auth0/auth0-acul-js/interstitial-captcha";
const interstitialCaptcha = new InterstitialCaptcha();
// SDK Properties return a string, number or boolean
// ex. "interstitial-captcha"
interstitialCaptcha.screen.name;
// SDK Methods return an object or array
// ex. { email: "someone@example.com" }
interstitialCaptcha.screenData();
Was this helpful?
/
Properties
The Interstitial Captcha screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
/
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
/
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
/
interface prompt{
name: string;
}
Was this helpful?
/
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | Record<string,
| string
| boolean
| string[]
| PhonePrefix[]>;
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
interface PhonePrefix {
country: string;
country_code: string;
phone_prefix: string;
}
Was this helpful?
/
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
/
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
/
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
/
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
/
Methods
The Interstitial Captcha screen class method is:
submitCaptcha( options? )
This method verifies the captcha response and directs the user to the next step.
interstitialCaptchaManager.submitCaptcha({
captcha: <CaptchaCodeResponse>
});
Was this helpful?
/
Parameter | Type | Required | Description |
---|---|---|---|
captcha |
string | Yes | The captcha code or response from the captcha provider. |
[key: string] |
string | number | boolean | undefined | No | Additional data collected from the user. |