Login screen classes
Login screen class
The Login screen class is part of the Identifier First Authentication flow and collects the user's identifier and password. Depending on your tenant setup, this identifier can be an email, phone number, or username.
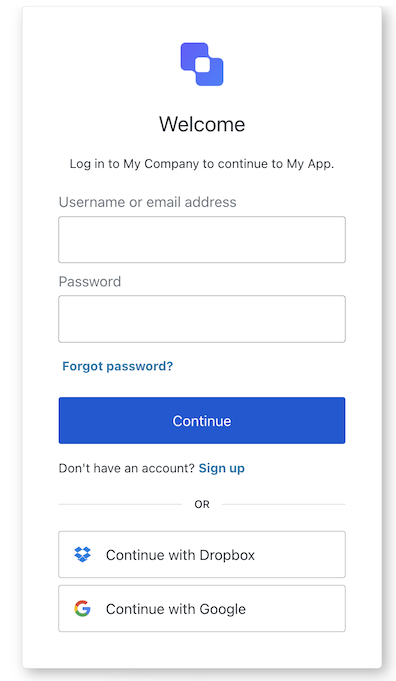
Import and instantiate the Login screen class:
import Login from "@auth0/auth0-acul-js/login";
const loginManager = new Login();
// SDK Properties return a string, number or boolean
// ex. "login-id"
loginManager.screen.name;
// SDK Methods return an object or array
// ex. { signup: "/signup_url", reset_password: "/reset_password_url"}
loginManager.screenLinks();
Was this helpful?
Properties
The Login screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | {username?: string;};
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
resetPasswordLink: null | string;
signupLink: null | string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
allowedIdentifiers: null | ("email" | "username" | "phone")[];
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
isForgotPasswordEnabled: boolean;
isPasskeyEnabled: boolean;
isSignupEnabled: boolean;
locale: string;
state: string;
getPasswordPolicy(): null | PasswordPolicy;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
interface PasswordPolicy {
minLength?: number;
policy:
| "low"
| "fair"
| "good"
| "excellent";
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The Login screen class methods are:
login( options? )
This method authenticates the user based on the identifier and provided password. Depending on the server configuration, this could be an email, username, or phone number. Review Flexible Identifiers for more details.
loginManager.login({
username: "testUser",
password: "testPassword"
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
username |
string | Yes | The user’s identifier. |
password |
string | Yes | The user's password. |
captcha |
string | Conditionally | The captcha code or response from the captcha provider. This property is required if your Auth0 tenant has Bot Detection enabled. |
[key: string] |
string | number | boolean | undefined | No | Additional data collected from the user. This data is accessible in the post-login Action trigger. |
socialLogin( options? )
This method redirects the user to the social or enterprise identity provider (IdP) for authentication. Review Social Identity Providers and Enterprise Identity Providers for details.
loginManager.socialLogin({
connection: "google-oauth2"
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
connection |
string | Yes | The identifier for the connection. |
[key: string] |
string | number | boolean | undefined | No | Additional data collected from the user. This data is accessible in the post-login Action trigger. |
Login Email Verification screen class
The Login Email Verification screen class provides methods associated with the login-email-verification screen.
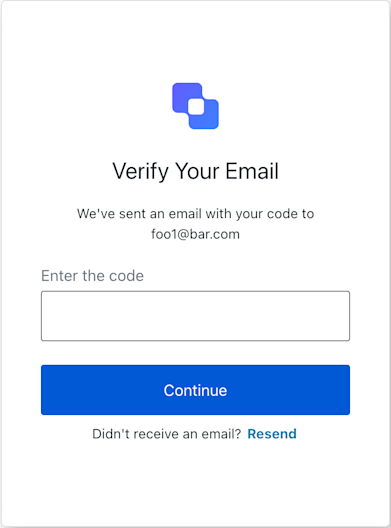
Import and instantiate the Login Email Verification screen class:
import LoginEmailVerification from '@auth0/auth0-acul-js/login-email-verification';
// Instantiate the manager for the login email verification screen
const loginEmailVerificationManager = new LoginEmailVerification();
// Accessing screen-specific texts (e.g., for titles, labels, button texts)
const screenTexts = loginEmailVerificationManager.screen.texts;
const pageTitle = screenTexts?.title || 'Verify Your Email';
const codePlaceholder = screenTexts?.codePlaceholder || 'Enter code here';
// Accessing transaction errors from a previous attempt
const transactionErrors = loginEmailVerificationManager.transaction.errors;
if (transactionErrors && transactionErrors.length > 0) {
transactionErrors.forEach(error => {
console.error(`Error Code: ${error.code}, Message: ${error.message}`);
// Display these errors to the user appropriately.
});
}
Was this helpful?
Properties
The Login Email Verification screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | Record<string,
| string
| boolean
| string[]
| PhonePrefix[]>
| Record<string, string[]>>;
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
interface PhonePrefix {
country: string;
country_code: string;
phone_prefix: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The Login Email Verification screen class methods are:
continueWithCode( options? )
This method submits the email verification code entered by the user to Auth0.
const manager = new LoginEmailVerification();
// Assuming 'userInputCode' is a string obtained from a form input
manager.continueWithCode({ code: userInputCode })
.catch(err => {
// Handle unexpected submission errors
displayGlobalError("Could not submit your code. Please try again.");
});
// After the operation, check manager.transaction.errors for validation messages.
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
code |
string | Yes | The code entered by the user. |
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
resendCode( options? )
This method requests Auth0 to send a new verification code to the user's email address.
const manager = new LoginEmailVerification();
manager.resendCode()
.then(() => {
// Inform the user that a new code has been sent.
showNotification("A new verification code is on its way!");
})
.catch(err => {
// Handle unexpected submission errors
displayGlobalError("Could not request a new code. Please try again later.");
});
// After the operation, check manager.transaction.errors for specific issues.
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
Login ID screen class
The Login ID screen class is part of the Identifier First Authentication flow and collects the user's identifier. Depending on your tenant setup, this identifier can be an email, phone number, or username.
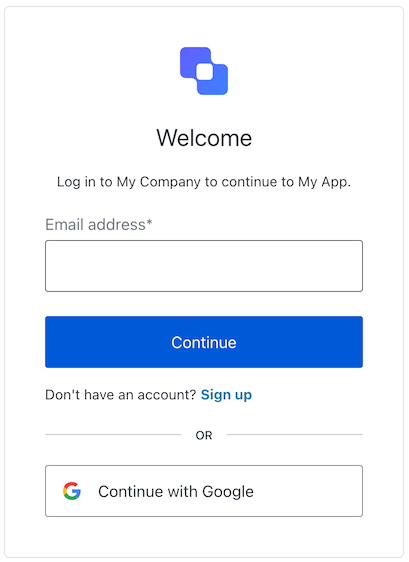
Import and instantiate the Login ID screen class:
import LoginId from '@auth0/auth0-acul-js/login-id';
const loginIdManager = new LoginId();
// SDK Properties return a string, number or boolean
// ex. "login-id"
loginIdManager.screen.name;
// SDK Methods return an object or array
// ex. { signup: "/signup_url", reset_password: "/reset_password_url"}
loginIdManager.screenLinks();
Was this helpful?
Properties
The Login ID screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | Record<string, string>;
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
publicKey: null | { challenge: string;};
resetPasswordLink: null | string;
signupLink: null | string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
allowedIdentifiers: null | ("email" | "username" | "phone")[];
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
isForgotPasswordEnabled: boolean;
isPasskeyEnabled: boolean;
isSignupEnabled: boolean;
isUsernameRequired: boolean;
locale: string;
state: string;
usernamePolicy: null | UsernamePolicy;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
display_name?: string;
icon_url?: string;
show_as_button: boolean;
};
strategy: string;
}
interface UsernamePolicy {
allowedFormats: {
usernameInEmailFormat: boolean;
usernameInPhoneFormat: boolean;
};
maxLength: number;
minLength: number;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The Login ID screen class methods are:
login( options? )
This method takes the user to the next step where they can enter their password or one-time password (OTP) code. Depending on the server configuration, this could be an email, username, or phone number. Review Flexible Identifiers for more details.
loginIdManager.login({
username: <identifierFieldValue>
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
username |
string | Yes | The user’s identifier. |
captcha |
string | Conditionally | The captcha code or response from the captcha provider. This property is required if your Auth0 tenant has Bot Detection enabled. |
[key: string] |
string | number | boolean | undefined | No | Additional data collected from the user. This data is accessible in the post-login Action trigger. |
passkeyLogin( options? )
This method authenticates the user using the provided passkey and, if successful, redirects them to the redirect_url
.
// This method does not support any parameters
loginIdManager.passkeyLogin();
Was this helpful?
socialLogin( options? )
This method redirects the user to the social or enterprise identity provider (IdP) for authentication. Review Social Identity Providers and Enterprise Identity Providers for details.
// Get the desired connection
const selectedConnection = alternateConnections.filter((connection) =>
connection.strategy === "google-oauth2"
});
loginIdManager.socialLogin({
connection: selectedConnection.strategy
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
connection |
string | Yes | The identifier for the connection. |
[key: string] |
string | number | boolean | undefined | No | Additional data collected from the user. This data is accessible in the post-login Action trigger. |
pickCountryCode( options? )
This method redirects the user to the country code selection list, where they can update the country code prefix for their phone number.
// This method does not support any parameters
loginIdManager.pickCountryCode();
Was this helpful?
Login Password screen class
The Login Password screen class is part of the Identifier First Authentication flow and collects the user's password.
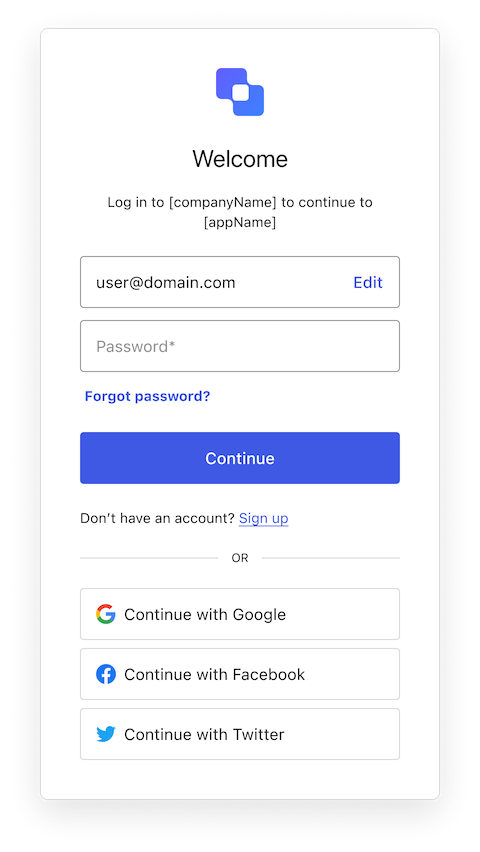
Import and instantiate the Login Password screen class
import LoginPassword from "@auth0/auth0-acul-js/login-password";
const loginPasswordManager = new LoginPassword();
// SDK Properties return a string, number or boolean
// ex. "login-password"
loginPasswordManager.screen.name;
// SDK Methods return an object or array
// ex. { signup: "/signup_url", reset_password: "/reset_password_url"}
loginPasswordManager.screenLinks();
Was this helpful?
Properties
The Login Password screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | { username: string; };
editIdentifierLink: null | string;
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
resetPasswordLink: null | string;
signupLink: null | string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
isForgotPasswordEnabled: boolean;
isPasskeyEnabled: boolean;
isSignupEnabled: boolean;
locale: string;
state: string;
getAllowedIdentifiers(): null | ("email" | "username" | "phone")[];
getPasswordPolicy(): null | PasswordPolicy;
getUsernamePolicy(): null | UsernamePolicy;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconURL?: string;
showAsButton: boolean;
};
strategy: string;
}
interface PasswordPolicy {
minLength?: number;
policy:
| "low"
| "fair"
| "good"
| "excellent";
}
interface UsernamePolicy {
allowedFormats: {
usernameInEmailFormat: boolean;
usernameInPhoneFormat: boolean;
};
maxLength: number;
minLength: number;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The Login Password screen class method is:
login( options? )
This method authenticates the user based on the identifier from the previous step and the provided password. Once authenticated, the user is directed to the next step.
loginPasswordManager.login({
username: <usernameFieldValue>,
password: ********
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
captcha |
string | Conditionally | The captcha code or response from the captcha provider. This property is required if your Auth0 tenant has Bot Detection enabled. |
username |
string | Yes | The user’s identifier. |
password |
string | Yes | The user's password. |
[key: string] |
string | number | boolean | undefined | No | Additional data collected from the user. This data is accessible in the post-login Action trigger. |
Login Passwordless Email Code screen class
The Login Passwordless Email Code screen class is part of the Identifier First Authentication flow. It allows the user to authenticate with a one-time password (OTP) code sent to the email provided in their previous step.
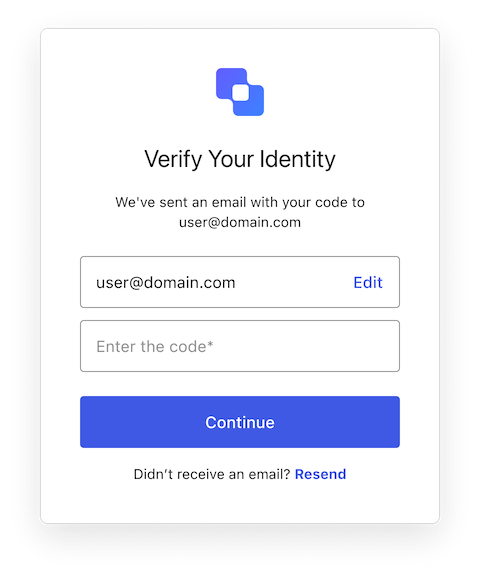
Import and instantiate the Login Passwordless Email Code screen class
import LoginPasswordlessEmailCode from '@auth0/auth0-acul-js/login-passwordless-email-code';
const loginPasswordlessEmailCodeManager = new LoginPasswordlessEmailCode();
// SDK Properties return a string, number or boolean
// ex. "login-passwordless-email-code"
loginPasswordlessEmailCodeManager.screen.name;
// SDK Methods return an object or array
// ex. { edit_identifier: "/edit_url" }
loginPasswordlessEmailCodeManager.screenLinks();
Was this helpful?
Properties
The Login Passwordless Email Code screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | {
email?: string;
username?: string;
};
editIdentifierLink: null | string;
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
resetPasswordLink: null | string;
signupLink: null | string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
isSignupEnabled: null | boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
display_name?: string;
icon_url?: string;
show_as_button: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The Login Passwordless Email Code screen class method are:
resendCode( options? )
This method sends a new OTP code to the email provided in the previous step.
// This method does not support any parameters
loginPasswordlessEmailCode.resendCode();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
SubmitCode( options? )
This method authenticates the user based on the provided email address and OTP code.
loginPasswordlessEmailCode.submitCode({
email: <EmailFieldValue>;
code: <OtpCodeFieldValue>;
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
captcha |
string | Conditionally | The captcha code or response from the captcha provider. This property is required if your Auth0 tenant has Bot Detection enabled. |
code |
string number | Yes | The OTP code sent to the email. |
[key: string] |
string | number | boolean | undefined | No | Additional data collected from the user. This data is accessible in the post-login Action trigger. |
Login Passwordless SMS OTP screen class
The Login Passwordless SMS OTP screen class is part of the Identifier First Authentication flow. It allows the user to authenticate with a one-time password (OTP) code sent to the phone number provided in the previous step.
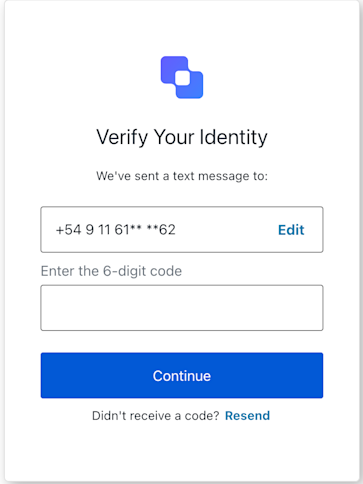
Import and instantiate the Login Passwordless Email Code screen class
import LoginPasswordlessSmsOtp from '@auth0/auth0-acul-js/login-passwordless-sms-otp';
const loginPasswordlessSmsOtpManager = new LoginPasswordlessSmsOtp();
// SDK Properties return a string, number or boolean
// ex. "login-passwordless-sms-otp"
loginPasswordlessSmsOtpManager.screen.name;
// SDK Methods return an object or array
// ex. { edit_identifier: "/edit_url" }
loginPasswordlessSmsOtpManager.screenLinks();
Was this helpful?
Properties
The Login Passwordless SMS OTP screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
backLink: null | string;
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: {username: string;};
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
resetPasswordLink: null | string;
signupLink: null | string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
isSignupEnabled: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
display_name?: string;
icon_url?: string;
show_as_button: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The Login Passwordless SMS OTP screen class method are:
resendOTP( options? )
This method sends a new OTP code to the email provided in the previous step.
// This method does not support any parameters
loginPasswordlessSmsOtpManager.resendOTP()
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
submitOTP( options? )
This method authenticates the user based on the provided phone number and OTP code.
loginPasswordlessSmsOtpManager.submitOTP({
username: <PhoneFieldValue>;
otp: <OtpCodeFieldValue>;
})
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
captcha |
string | Conditionally | The captcha code or response from the captcha provider. This property is required if your Auth0 tenant has Bot Detection enabled. |
otp |
string | Yes | The OTP code sent to the phone number. |
username |
string | Yes | The phone number from the previous step. |
[key: string] |
string | number | boolean | undefined | No | Optional data collected from the user. |