Multi-Factor Authentication Push screen classes
MFA Push Challenge Push screen class
The MFA Push Challenge Push screen class is displayed when a push notification has been sent to user's device.
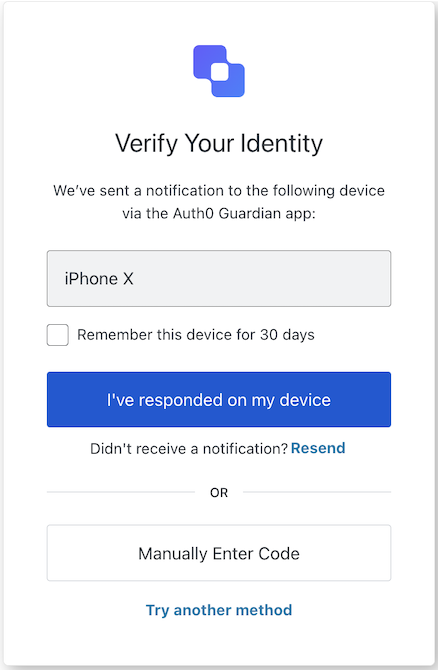
Import and instantiate the MFA Push Challenge Push screen class:
import MfaPushChallengePush from '@auth0/auth0-acul-js/mfa-push-challenge-push';
const mfaPushChallengePush = new MfaPushChallengePush();
// SDK Properties return a string, number or boolean
mfaPushChallengePush.screen.name;
// SDK Methods return an object or array
await mfaPushChallengePush.continue();
Was this helpful?
Properties
The MFA Push Challenge Push screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | {
deviceName: string;
rememberDevice?: boolean
};
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The MFA Push Challenge Push screen class method are:
continue( options ?)
This method continues with the push notification challenge.
const mfaPushChallengePush = new MfaPushChallengePush();
await mfaPushChallengePush.continue();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
enterCodeManually( options ?)
This method allows the user to enter the verification code manually.
const mfaPushChallengePush = new MfaPushChallengePush();
await mfaPushChallengePush.enterCodeManually();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
resendPushNotification( options ?)
This method resends the push notification.
const mfaPushChallengePush = new MfaPushChallengePush();
await mfaPushChallengePush.resendPushNotification();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
tryAnotherMethod( options ?)
This method allows the user to try another authentication method.
const mfaPushChallengePush = new MfaPushChallengePush();
await mfaPushChallengePush.tryAnotherMethod();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
MFA Push Enrollment QR screen class
The MFA Push Enrollment QR screen class implements the scan QR code screen.
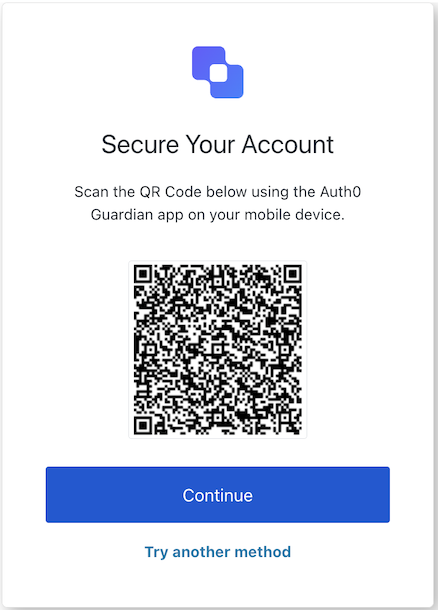
Import and instantiate the MFA Push Enrollment QR screen class:
import MfaPushEnrollmentQr from '@auth0/auth0-acul-js/mfa-push-enrollment-qr';
const mfaPushEnrollmentQr = new MfaPushEnrollmentQr();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaPushEnrollmentQr.screen.name;
// SDK Methods return an object or array
await mfaPushEnrollmentQr.pickAuthenticator();
Was this helpful?
Properties
The MFA Push Enrollment QR screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | {qr_code: string;};
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The MFA Push Enrollment QR screen class method is:
pickAuthenticator( options ?)
This method navigates the user to the authenticator selection screen.
import MfaPushEnrollmentQr from '@auth0/auth0-acul-js/mfa-push-enrollment-qr';
const mfaPushEnrollmentQr = new MfaPushEnrollmentQr();
await mfaPushEnrollmentQr.pickAuthenticator();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
MFA Push List screen class
The MFA Push List screen class implements the mfa-push-list screen functionality.
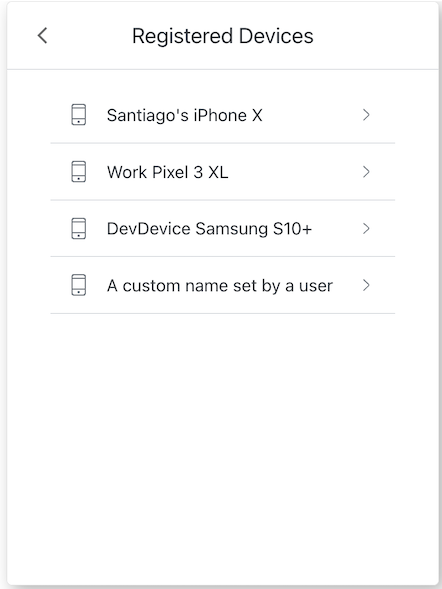
Import and instantiate the MFA Push List screen class:
import MfaPushList from '@auth0/auth0-acul-js/mfa-push-list';
const mfaPushList = new MfaPushList();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaPushList.screen.name;
// SDK Methods return an object or array
await mfaPushList.selectMfaPushDevice({ deviceIndex: 0 });
Was this helpful?
Properties
The MFA Push List screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | {enrolled_devices: string[];};
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The MFA Push List screen class method are:
goBack( options ?)
This method allows the user to navigate to previous screen.
import MfaPushList from '@auth0/auth0-acul-js/mfa-push-list';
const mfaPushList = new MfaPushList();
await mfaPushList.goBack();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
selectMfaPushDevice( options ?)
This method allows the user to select from a list of registered device to initiate MFA push.
import MfaPushList from '@auth0/auth0-acul-js/mfa-push-list';
const mfaPushList = new MfaPushList();
await mfaPushList.selectMfaPushDevice({ deviceIndex: 0 });
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
deviceIndex |
number | Yes | The index of the device to select from the list of enrolled devices. |
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
MFA Push Welcome screen class
The MFA Push Welcome screen class initializes the MfaPushWelcome screen with data from the Universal Login Context.
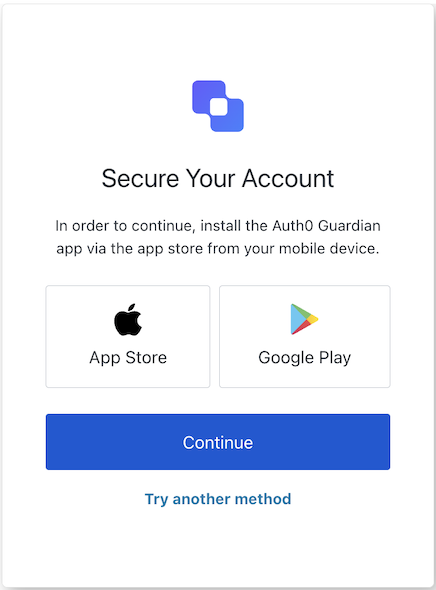
Import and instantiate the MFA Push Welcome screen class:
import MfaPushWelcome from '@auth0/auth0-acul-js/mfa-push-welcome';
const mfaPushWelcome = new MfaPushWelcome();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaPushWelcome.screen.name;
// SDK Methods return an object or array
await mfaPushWelcome.enroll();
Was this helpful?
Properties
The MFA Push Welcome screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | Record<string,
| string
| boolean
| string[]
| PhonePrefix[]>;
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
screen: {
links: {
android: string;
ios: string;
};
name: string;
};
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
interface PhonePrefix {
country: string;
country_code: string;
phone_prefix: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The MFA Push Welcome screen class method are:
enroll( options ?)
This method navigates the user to the enrollment screen.
import MfaPushWelcome from '@auth0/auth0-acul-js/mfa-push-welcome';
const mfaPushWelcome = new MfaPushWelcome();
await mfaPushWelcome.enroll();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
pickAuthenticator( options ?)
This method navigates the user to the authenticator selection screen.
import MfaPushWelcome from '@auth0/auth0-acul-js/mfa-push-welcome';
const mfaPushWelcome = new MfaPushWelcome();
await mfaPushWelcome.pickAuthenticator();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |