Multi-Factor Authentication SMS screen classes
MFA SMS Challenge screen class
The MFA SMS Challenge screen class provides methods associated with the mfa-sms-challenge screen.
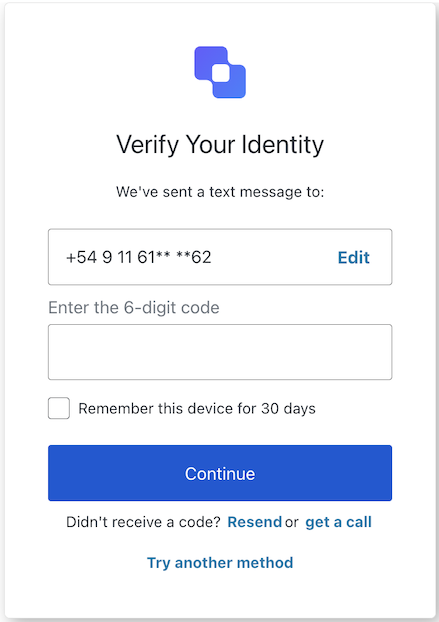
Import and instantiate the MFA SMS Challenge screen class:
import MfaSmsChallenge from '@auth0/auth0-acul-js/mfa-sms-challenge';
const mfaSmsChallenge = new MfaSmsChallenge();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaSmsChallenge.screen.name;
// SDK Methods return an object or array
await mfaSmsChallenge.continueMfaSmsChallenge({
code: '123456',
rememberBrowser: true,
});
Was this helpful?
Properties
The MFA SMS Challenge screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | {
phone_number?: string;
remember_device?: boolean;
};
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The MFA SMS Challenge screen class method are:
continueMfaSmsChallenge( options ?)
This method submits the entered verification code and rememberBrowser option.
import MfaSmsChallenge from '@auth0/auth0-acul-js/mfa-sms-challenge';
const mfaSmsChallenge = new MfaSmsChallenge();
await mfaSmsChallenge.continueMfaSmsChallenge({
code: '123456',
rememberBrowser: true,
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
code |
string | Yes | The code entered by the user. |
rememberBrowser |
boolean | No | Remember the user's browser configuration. |
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
getACall( options ?)
This method allows to send the verification code by voice call verification.
import MfaSmsChallenge from '@auth0/auth0-acul-js/mfa-sms-challenge';
const mfaSmsChallenge = new MfaSmsChallenge();
await mfaSmsChallenge.getACall();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
pickSms( options ?)
This methods allows the user to select a different SMS configuration, if available.
import MfaSmsChallenge from '@auth0/auth0-acul-js/mfa-sms-challenge';
const mfaSmsChallenge = new MfaSmsChallenge();
await mfaSmsChallenge.pickSms();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
resendCode( options ?)
This methods resends the SMS code.
import MfaSmsChallenge from '@auth0/auth0-acul-js/mfa-sms-challenge';
const mfaSmsChallenge = new MfaSmsChallenge();
await mfaSmsChallenge.resendCode();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
tryAnotherMethod( options ?)
This method submits the action to try another MFA method.
import MfaSmsChallenge from '@auth0/auth0-acul-js/mfa-sms-challenge';
const mfaSmsChallenge = new MfaSmsChallenge();
await mfaSmsChallenge.tryAnotherMethod();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
MFA SMS Enrollment screen class
The MFA SMS Enrollment screen class provides methods associated with the mfa-sms-challenge screen.
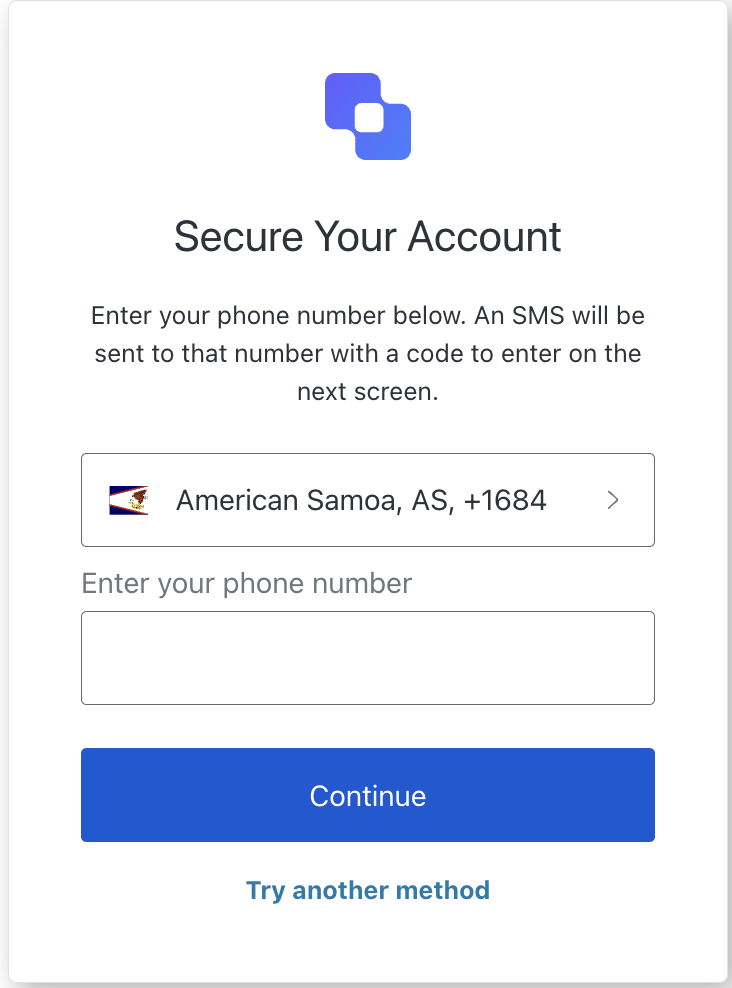
Import and instantiate the MFA SMS Enrollment screen class:
import MfaSmsEnrollment from '@auth0/auth0-acul-js/mfa-sms-enrollment';
const mfaSmsEnrollment = new MfaSmsEnrollment();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaSmsEnrollment.screen.name;
// SDK Methods return an object or array
await mfaSmsEnrollment.pickCountryCode();
Was this helpful?
Properties
The MFA SMS Enrollment screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | {phone?: string;};
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The MFA SMS Enrollment screen class method are:
continueEnrollment( options ?)
This method continues the SMS enrollment process with the provided phone number.
import MfaSmsEnrollment from '@auth0/auth0-acul-js/mfa-sms-enrollment';
const mfaSmsEnrollment = new MfaSmsEnrollment();
await mfaSmsEnrollment.continueEnrollment({ phone: '1234567890' });
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
captcha |
string | Conditionally | The captcha code or response from the captcha provider. |
phone |
string | No | The phone number entered by the user. |
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
pickCountryCode( options ?)
This method handles the action to select a country code for SMS enrollment.
import MfaSmsEnrollment from '@auth0/auth0-acul-js/mfa-sms-enrollment';
const mfaSmsEnrollment = new MfaSmsEnrollment();
await mfaSmsEnrollment.pickCountryCode();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
tryAnotherMethod( options ?)
This method handles the action to try another method for MFA.
import MfaSmsEnrollment from '@auth0/auth0-acul-js/mfa-sms-enrollment';
const mfaSmsEnrollment = new MfaSmsEnrollment();
await mfaSmsEnrollment.tryAnotherMethod();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
MFA SMS List screen class
The MFA SMS List screen class provides methods associated with the mfa-sms-list screen.
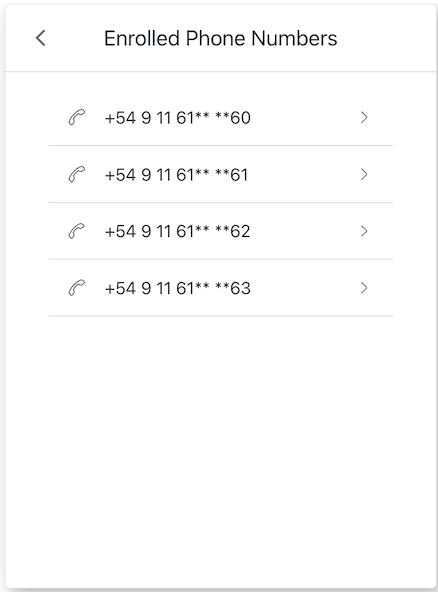
Import and instantiate the MFA SMS List screen class:
import MfaSmsList from '@auth0/auth0-acul-js/mfa-sms-list';
const mfaSmsList = new MfaSmsList();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaSmsList.screen.name;
// SDK Methods return an object or array
// ex. { signup: "/signup_url", reset_password: "/reset_password_url"}
await mfaSmsList.backAction();
Was this helpful?
Properties
The MFA SMS List class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | Record<string,
| string
| boolean
| string[]
| PhonePrefix[]>
| Record<string, string[]>>;
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
interface PhonePrefix {
country: string;
country_code: string;
phone_prefix: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The MFA SMS List screen class method are:
backAction( options ?)
This method allows the user to navigate to the previous screen.
import MfaSmsList from '@auth0/auth0-acul-js/mfa-sms-list';
const mfaSmsList = new MfaSmsList();
await mfaSmsList.backAction();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
selectPhoneNumber( options ?)
This method allows the user to select a phone number from the list of enrolled phone numbers.
import MfaSmsList from '@auth0/auth0-acul-js/mfa-sms-list';
const mfaSmsList = new MfaSmsList();
await mfaSmsList.selectPhoneNumber({
index: 0 // for demonstration we are selecting the first index
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
index |
number | Yes | The index of the Phone number to select. |
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
MFA Country Codes screen class
The MFA Country Codes screen class allows users to select a country code for MFA phone number verification.
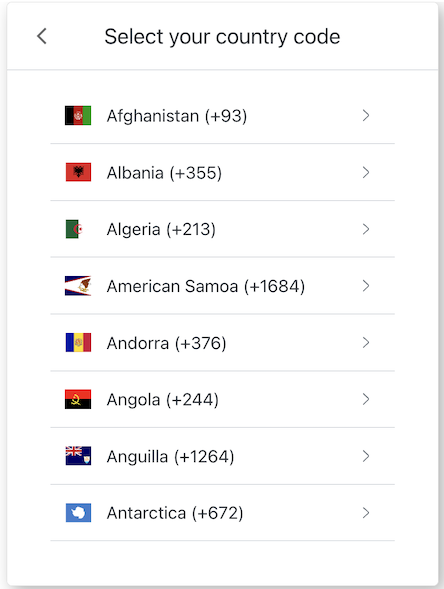
Import and instantiate the MFA Country Codes screen class:
import MfaCountryCodes from '@auth0/auth0-acul-js/mfa-country-codes';
const mfaCountryCodesManager = new MfaCountryCodes();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaCountryCodesManager.screen.name;
// SDK Methods return an object or array
// ex. { signup: "/signup_url", reset_password: "/reset_password_url"}
await mfaCountryCodesManager.selectCountryCode({
action: 'selection-action::US1'
});
Was this helpful?
Properties
The MFA Country Codes screen class properties are:
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | { phone_prefixes: PhonePrefix[]; };
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
interface PhonePrefix {
country: string;
country_code: string;
phone_prefix: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
Methods
The MFA Country Codes screen class method are:
goBack( options ?)
This method navigates the user to the previous screen.
import MfaCountryCodes from '@auth0/auth0-acul-js/mfa-country-codes';
const mfaCountryCodes = new MfaCountryCodes();
await mfaCountryCodes.goBack();
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |
selectCountryCode( options ?)
This method allows the user to select a country code.
import MfaCountryCodes from '@auth0/auth0-acul-js/mfa-country-codes';
const mfaCountryCodes = new MfaCountryCodes();
// Get the available country codes and phone prefixes
const { screen } = mfaCountryCodes;
const { phone_prefixes } = screen.data
const {country_code, phone_prefix} = phone_prefixes[0]
await mfaCountryCodes.selectCountryCode({
country_code: 'US',
phone_prefix: '+1',
});
Was this helpful?
Parameter | Type | Required | Description |
---|---|---|---|
country_code |
string | Yes | Country code to select. |
phone_prefix |
string | Yes | Phone prefix to select. |
[key: string] |
string | number | boolean | undefined | No | Optional data collected from user. |