多要素認証メール画面クラス
MFAメールチャレンジ画面クラス
MFAメールチャレンジ画面クラスは、ユーザーがMFAを使用して自身のメールアドレスを確認できるようにします。
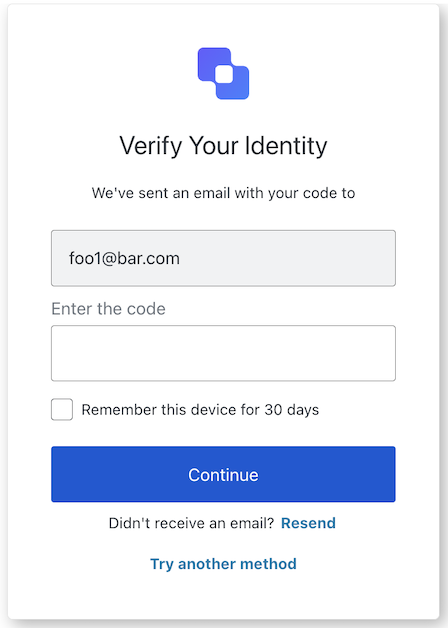
MFAメールチャレンジ画面クラスのインポートとインスタンス化
import MfaEmailChallenge from '@auth0/auth0-acul-js/mfa-email-challenge';
const mfaEmailChallengeManager = new MfaEmailChallenge();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaDetectBrowserCapabilitiesManager.screen.name;
// SDK Methods return an object or array
// ex. { signup: "/signup_url", reset_password: "/reset_password_url"}
await mfaEmailChallenge.continue({
code: '123456',
rememberDevice: true
});
Was this helpful?
プロパティ
MFAメールチャレンジ画面クラスには以下のプロパティがあります。
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | {
email: string;
remember_device?: boolean;
};
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
メソッド
MFAメールチャレンジ画面クラスには以下のメソッドがあります。
continue( options ?)
このメソッドは提供されたコードを使用してメールチャレンジを続行します。
import MfaEmailChallenge from '@auth0/auth0-acul-js/mfa-email-challenge';
const mfaEmailChallenge = new MfaEmailChallenge();
await mfaEmailChallenge.continue({
code: '123456',
rememberDevice: true
});
Was this helpful?
パラメーター | タイプ | 必須 | 説明 |
---|---|---|---|
code |
文字列 | 必須 | ユーザーが入力したコードです。 |
rememberDevice |
ブール値 | 任意 | ユーザーのデバイスを記憶します。 |
[key: string] |
文字列 | 数値 | ブール値 | undefined | 任意 | ユーザーから収集した任意のデータです。 |
pickEmail( options ?)
このメソッドはユーザーが利用可能な別のメールを選択できるようにします。
import MfaEmailChallenge from '@auth0/auth0-acul-js/mfa-email-challenge';
const mfaEmailChallenge = new MfaEmailChallenge();
await mfaEmailChallenge.pickEmail();
Was this helpful?
パラメーター | タイプ | 必須 | 説明 |
---|---|---|---|
[key: string] |
文字列 | 数字 | ブール値 | undefined | 任意 | ユーザーから収集した任意のデータです。 |
resendCode( options ?)
このメソッドはメールコードを再度送信します。
import MfaEmailChallenge from '@auth0/auth0-acul-js/mfa-email-challenge';
const mfaEmailChallenge = new MfaEmailChallenge();
await mfaEmailChallenge.resendCode();
Was this helpful?
パラメーター | タイプ | 必須 | 説明 |
---|---|---|---|
[key: string] |
文字列 | 数字 | ブール値 | undefined | 任意 | ユーザーから収集した任意のデータです。 |
tryAnotherMethod( options ?)
このメソッドはユーザーが別のMFAオプションを選択できるようにします。
import MfaEmailChallenge from '@auth0/auth0-acul-js/mfa-email-challenge';
const mfaEmailChallenge = new MfaEmailChallenge();
await mfaEmailChallenge.tryAnotherMethod();
Was this helpful?
パラメーター | タイプ | 必須 | 説明 |
---|---|---|---|
[key: string] |
文字列 | 数字 | ブール値 | undefined | 任意 | ユーザーから収集した任意のデータです。 |
MFAメールリスト画面クラス
MFAメールリスト画面クラスは、ユーザーがMFAに登録済みのメールアドレスを選択できるようにします。
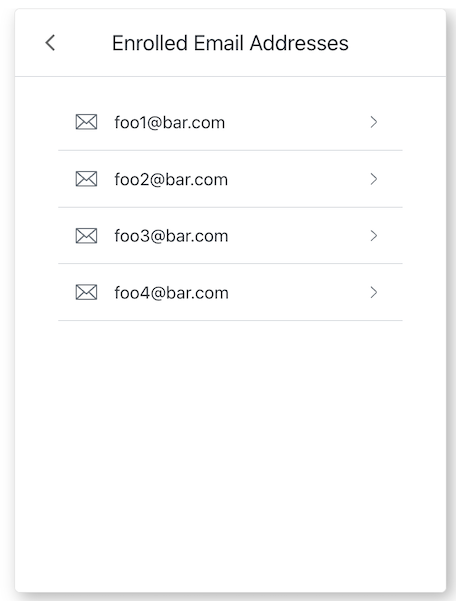
MFAメールリスト画面クラスのインポートとインスタンス化
import MfaEmailList from '@auth0/auth0-acul-js/mfa-email-list';
const mfaEmailList = new MfaEmailList();
// SDK Properties return a string, number or boolean
// ex. "login-id"
mfaEmailList.screen.name;
// SDK Methods return an object or array
await mfaEmailList.selectMfaEmail({
index: 0 // for demonstration we are selecting the first index
});
Was this helpful?
プロパティ
MFAメールリスト画面クラスには以下のプロパティがあります。
interface branding {
settings: null | BrandingSettings;
themes: null | BrandingThemes;
}
interface BrandingSettings {
colors?: {
pageBackground?: string | {
angleDeg: number;
end: string;
start: string;
type: string;
};
primary?: string;
};
faviconUrl?: string;
font?: {url: string;};
logoUrl?: string;
}
interface BrandingThemes {
default: {
borders: Record<string, string | number | boolean>;
colors: Record<string, string>;
displayName: string;
fonts: Record<string, string | boolean | object>;
pageBackground: Record<string, string>;
widget: Record<string, string | number>;
};
}
Was this helpful?
interface client {
description: null | string;
id: string;
logoUrl: null | string;
name: string;
metadata: null | {[key: string]: string;};
}
Was this helpful?
interface organization {
branding: null | {
colors?: {
pageBackground?: string;
primary?: string;
};
logoUrl?: string;
};
displayName: null | string;
id: null | string;
metadata: null | {[key: string]: string;};
name: null | string;
usage: null | string;
}
Was this helpful?
interface prompt{
name: string;
}
Was this helpful?
interface screen {
captcha: null | CaptchaContext;
captchaImage: null | string;
captchaProvider: null | string;
captchaSiteKey: null | string;
data: null | Record<string,
| string
| boolean
| string[]
| PhonePrefix[]>
| Record<string, string[]>>;
isCaptchaAvailable: boolean;
links: null | Record<string, string>;
name: string;
texts: null | Record<string, string>;
}
interface CaptchaContext {
image?: string;
provider: string;
siteKey?: string;
}
interface PhonePrefix {
country: string;
country_code: string;
phone_prefix: string;
}
Was this helpful?
interface tenant {
enabledFactors: null | string[];
enabledLocales: null | string[];
friendlyName: null | string;
name: null | string;
}
Was this helpful?
interface transaction {
alternateConnections: null | (Connection | EnterpriseConnection)[];
connectionStrategy: null | string;
countryCode: null | string;
countryPrefix: null | string;
currentConnection: null | Connection;
errors: null | Error[];
hasErrors: boolean;
locale: string;
state: string;
}
interface Connection {
metadata?: Record<string, string>;
name: string;
strategy: string;
}
interface EnterpriseConnection {
metadata?: Record<string, string>;
name: string;
options: {
displayName?: string;
iconUrl?: string;
showAsButton: boolean;
};
strategy: string;
}
Was this helpful?
interface untrustedData {
authorizationParams: null | {
login_hint?: string;
screen_hint?: string;
ui_locales?: string;
[key: `ext-${string}`]: string;
};
submittedFormData: null | {
[key: string]:
| string
| number
| boolean
| undefined;
};
}
Was this helpful?
interface user {
appMetadata: null | {[key: string]: string;};
email: null | string;
enrolledDevices: null | ShortEntity<"device">[];
enrolledEmails: null | ShortEntity<"email">[];
enrolledFactors: null | string[];
enrolledPhoneNumbers: null | ShortEntity<"phoneNumber">[];
id: null | string;
organizations: null | {
branding: undefined | {logoUrl: undefined | string;};
displayName: undefined | string;
organizationId: undefined | string;
organizationName: undefined | string;
}[];
phoneNumber: null | string;
picture: null | string;
userMetadata: null | {[key: string]: string;};
username: null | string;
}
ShortEntity<Key>: {
id: number;
} & Record<Key, string>
Was this helpful?
メソッド
MFAメールリスト画面クラスには以下のメソッドがあります。
goBack( options ?)
このメソッドはユーザーが前の画面に戻れるようにします。
import MfaEmailList from '@auth0/auth0-acul-js/mfa-email-list';
const mfaEmailList = new MfaEmailList();
await mfaEmailList.goBack();
Was this helpful?
パラメーター | タイプ | 必須 | 説明 |
---|---|---|---|
[key: string] |
文字列 | 数字 | ブール値 | undefined | 任意 | ユーザーから収集した任意のデータです。 |
selectMfaEmail( options ?)
このメソッドは、ユーザーが登録済みのメールアドレスのリストから選択できるようにします。
import MfaEmailList from '@auth0/auth0-acul-js/mfa-email-list';
const mfaEmailList = new MfaEmailList();
await mfaEmailList.selectMfaEmail({
index: 0 // for demonstration we are selecting the first index
});
Was this helpful?
パラメーター | タイプ | 必須 | 説明 |
---|---|---|---|
index |
数値 | 必須 | 選択するメールアドレスのインデックスです。 |
[key: string] |
文字列 | 数値 | ブール値 | undefined | 任意 | ユーザーから収集した任意のデータです。 |